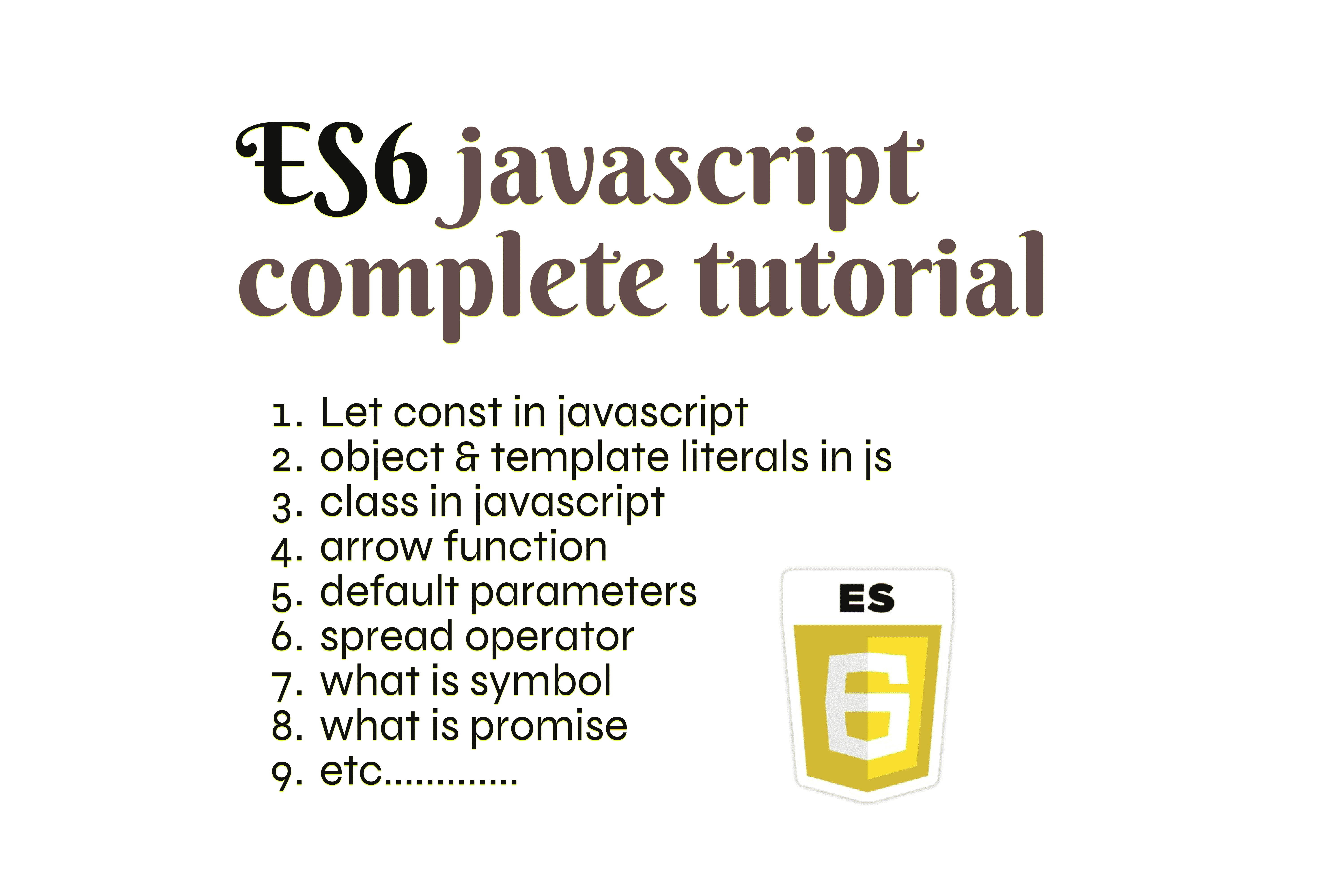
In this tutorial we're going to learn about javascript es6 concepts in depth like const let , symbol, promise , default parameters, object & template literals etc..
🔗Arrow function with this
🔗What is symbol ?
A JavaScript Symbol is a primitive datatype just like Number, String, or Boolean.
It represents a unique "hidden" identifier that no other code can accidentally access.
Create a symbol :-
the Symbol() function creates a new unique value each time you call it:
jslet s = Symbol("foo");console.log(Symbol() === Symbol()); // false
The Symbol() function accepts a description as an optional argument.
The description argument will make your symbol more descriptive.
The following example creates one symbols: firstName
jslet firstName = Symbol("first name");
You can access the symbol’s description property using the toString() method.
jsconsole.log(firstName.toString()); // Symbol(first name)

Symbols are primitive values, you can use the typeof operator to check primitive type
jsconsole.log(typeof firstName); // symbol

If you attempt to create a symbol using the new operator, you will get an error because symbol is a primitive value,
jslet newS = new Symbol();console.log(newS); // error
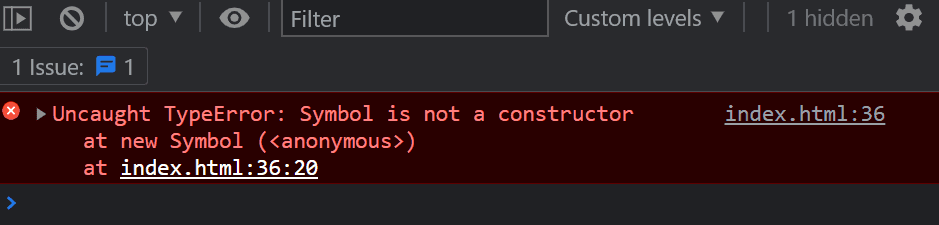
Symbol usages :-
Eg if different coders want to add a person.id property to a person object belonging to a third - party code, they could mix each others values.
Using Symbol() to create a unique identifiers, solves this problem:
jsconst person = {firstName: "John",lastName: "Doe",age: 50,eyeColor: "blue",};let id = Symbol("id");person[id] = 100123;console.log(person[id]); // 100123

🔗Let const
🔗What is promise ?
Is just like a promise in real life what you do is you commit to something by saying I promise to do something for example I promise to make the tutorial post on es6 then that promise either has two results either that promise has completed it is resolved or that promise is failed and it is rejected
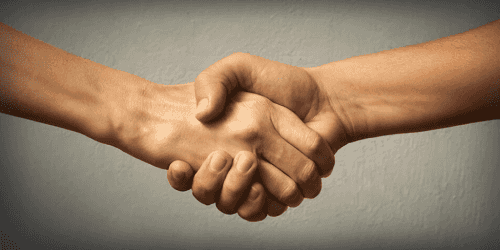
Similrarly in javascript we have promise , which contain producing code and consuming code
Producing code : is code that can take some time (define resolved and reject in promise)
Consuming code : is code that must wait for the result (result of promise completed or not)
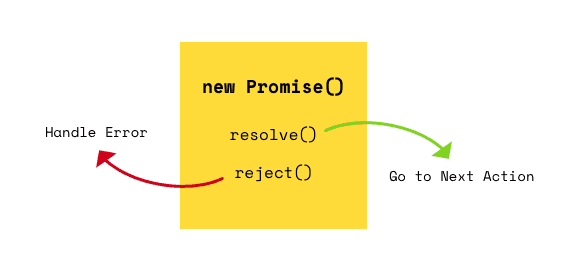
Create a Promise :-
To create a promise
object, we use the promise()
constructor.
jslet promise = new Promise(function (resolve, reject) {//do something});
The Promise()
constructor takes a function as an argument. The function accepts
two functions resolve() and reject(). If the promise returns successfully, the resolve()
function is called.And, if an error occurs, the reject()
function is called.
jslet promise = new Promise(function (resolve, reject) {let x = 0;if (x == 0) {resolve("success");} else {reject("Failed");}});
How to use promise
Promise.then() method takes two arguments, a callback for success and another for failure.
jspromise.then(function (value) {console.log(value);},function (error) {console.log(error);});
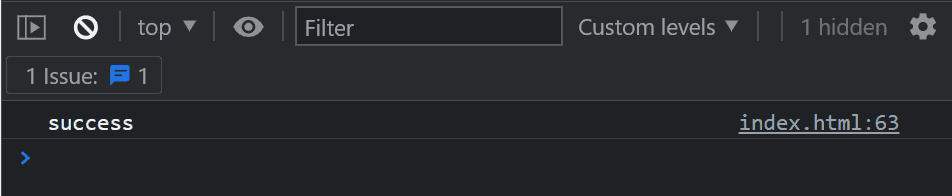