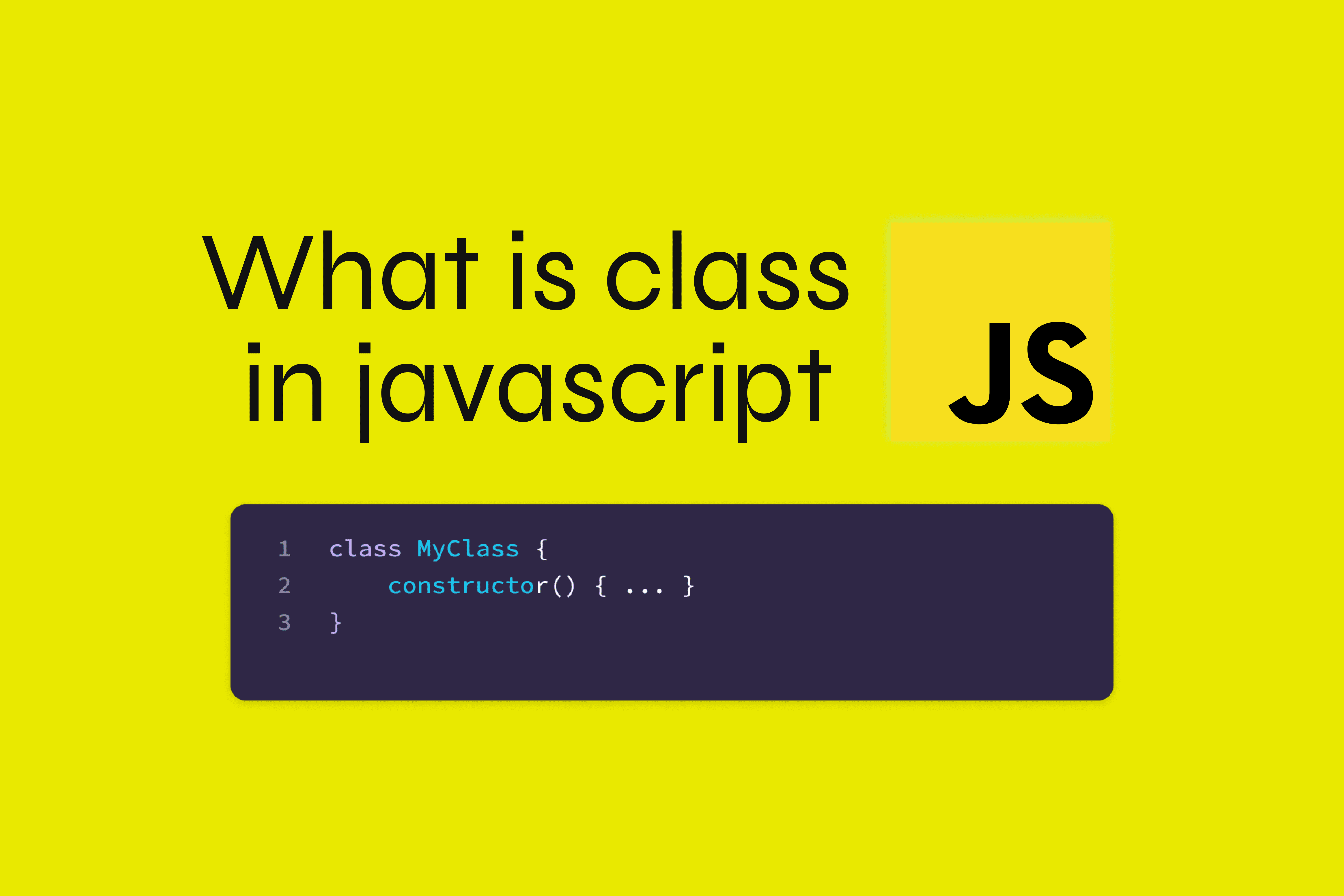
๐What is class ?
JavaScript Classes are templates for JavaScript Objects. class encapsulates data and functions that manipulate data
**syntax**
jsclass MyClass {constructor() { ... }}
Define a class :- in this example we created a class named "User", with the two properties "name" and "country".
jsclass User {constructor(name, country) {this.name = name;this.country = country;}}
Using a Class :- When you have a class, you can use the class to create objects:
jslet user1 = new User("Sumit", "India");console.log(`Hello i am ${user1.name} from ${user1.country}`);
The class Method :- in this example we created a class named "myAge", with properties "year" and age() method.
jsclass myAge {constructor(year) {this.year = year;}age() {return year - this.year;}}let date = new Date();let year = date.getFullYear();let howold = new myAge(2001);console.log("I'm" + " " + howold.age() + " years old.");// I'm 20 years old.
๐What is Class Inheritance ?
Class inheritance is a way for one class to extend another class using extend keyword and super method
Inheritance is useful for code reusability reuse properties and methods of an existing class when you create a new class.
Parents class
jsclass Car {constructor(brand) {this.carname = brand;}present() {return "I have a " + this.carname;}}
Extending Model class to parent car class
jsclass Model extends Car {constructor(brand, mod) {// super method to get access of parents class properties and methodsuper(brand);this.model = mod;}show() {return this.present() + ", it is a " + this.model;}}let myCar = new Model("THAR", "AX Opt");console.log(myCar.show());
