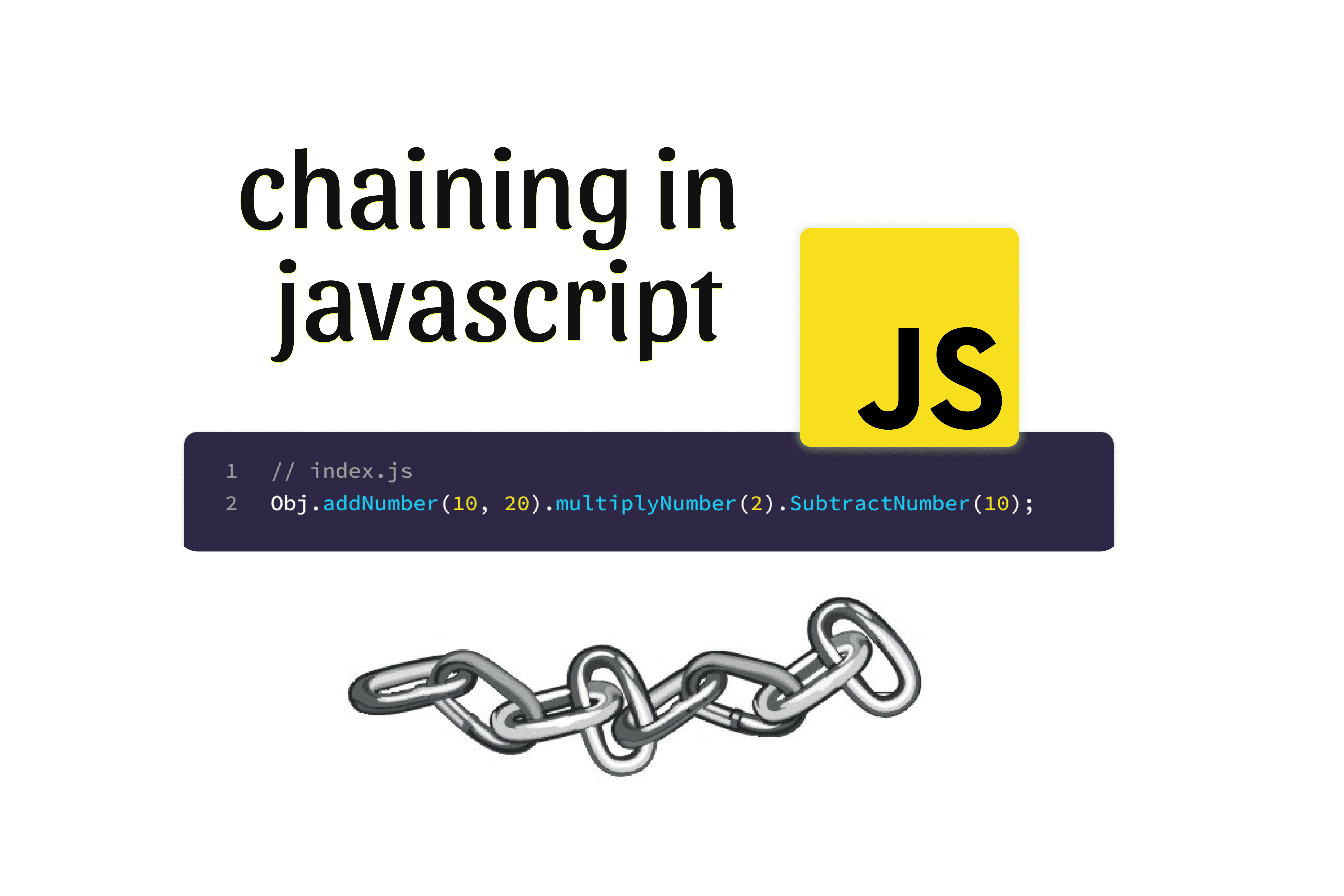
In this post weโll going to learn chaining
with 2 different examples 1 will be very simple to understand concept of chaining 2nd will be little bit complicated
๐What is Chaining ?
JavaScript allows you to invoke multiple methods
on an object in a single expression. Chaining is a process of stringing the method calls together with dots between them.
Syntax:-
jsobject.method1().method2().method3();
๐1 ) Example
In this simple example we have a class with 3 different methods each method console log a some string we invoked all 3 method on an object in a single expression using chaining
jsclass method_chaining {method1() {console.log("method1");return this;}method2() {console.log("method2");return this;}method3() {console.log("method3");return this;}}const method_chaining_obj = new method_chaining();method_chaining_obj.method1().method2().method3();
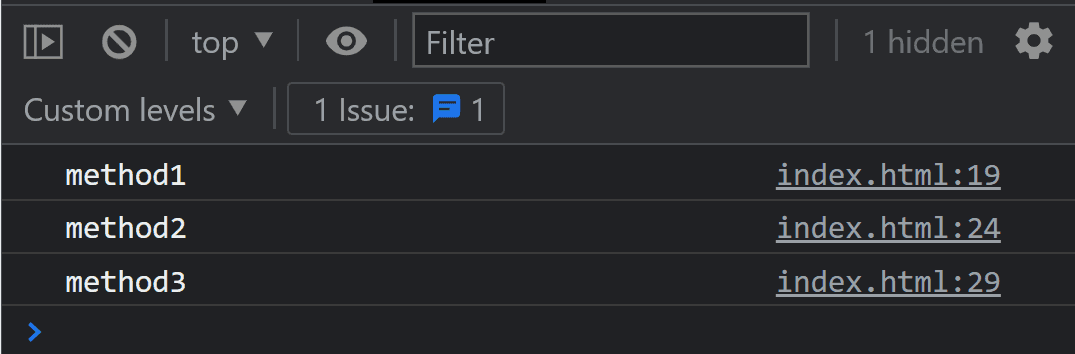
๐2) Example
Let's consider the following requirements and implement chaining for them
- Create a variable Obj containing a variable result & create function to add the variables and assign it to result
jsvar Obj = {result: 0,addNumber(a, b) {this.result = a + b;},};Obj.addNumber(10, 20); // 10 + 20 = 30console.log(Obj.result); // 30
The above object contains a single mathematical function to add a two numbers
We need to further extend the functionality to have multiple function chained together let's add one more functionality multiplyNumber
to be like this: ๐
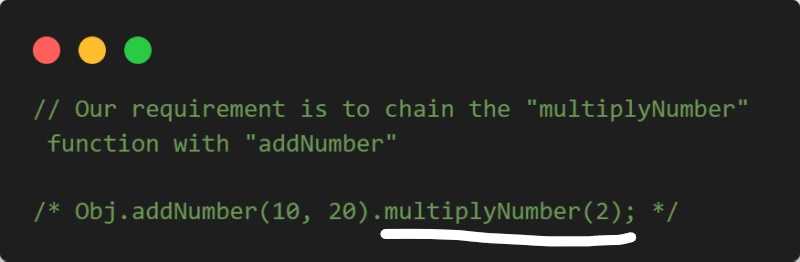
- function to multiply the result by 2
we have to return the current reference of the object that the function is currently executing in this refers to the current executing context let's try using this
Using a this
to return the current object reference from the addNumber
function
jsvar Obj = {result: 0,addNumber: function (a, b) {this.result = a + b;return this;},multiplyNumber: function (a) {this.result = this.result * a;return this;},};Obj.addNumber(10, 20).multiplyNumber(2); // 10+20 = 30 * 2 = 60console.log(Obj.result); // 60
In the above code, the function addNumber returns the current executing context back from the function call. The next function then executes on this context (referring to the same object), and invokes the other functions associated with the object.
- function to Subtract 10 from it and display the result
jsvar Obj = {result: 0,addNumber: function (a, b) {this.result = a + b;return this;},multiplyNumber: function (a) {this.result = this.result * a;return this;},SubtractNumber: function (a) {this.result = this.result - a;return this;},};
Hence were able to achieve function chaining.
jsObj.addNumber(10, 20).multiplyNumber(2).SubtractNumber(10);// 10+20 = 30 * 2 = 60 - 10 = 50console.log(Obj.result); // 50
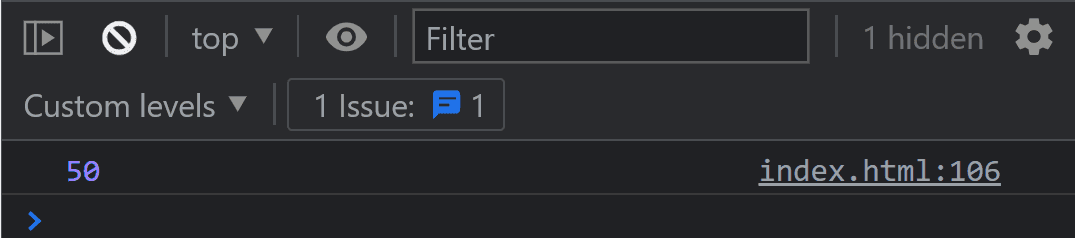