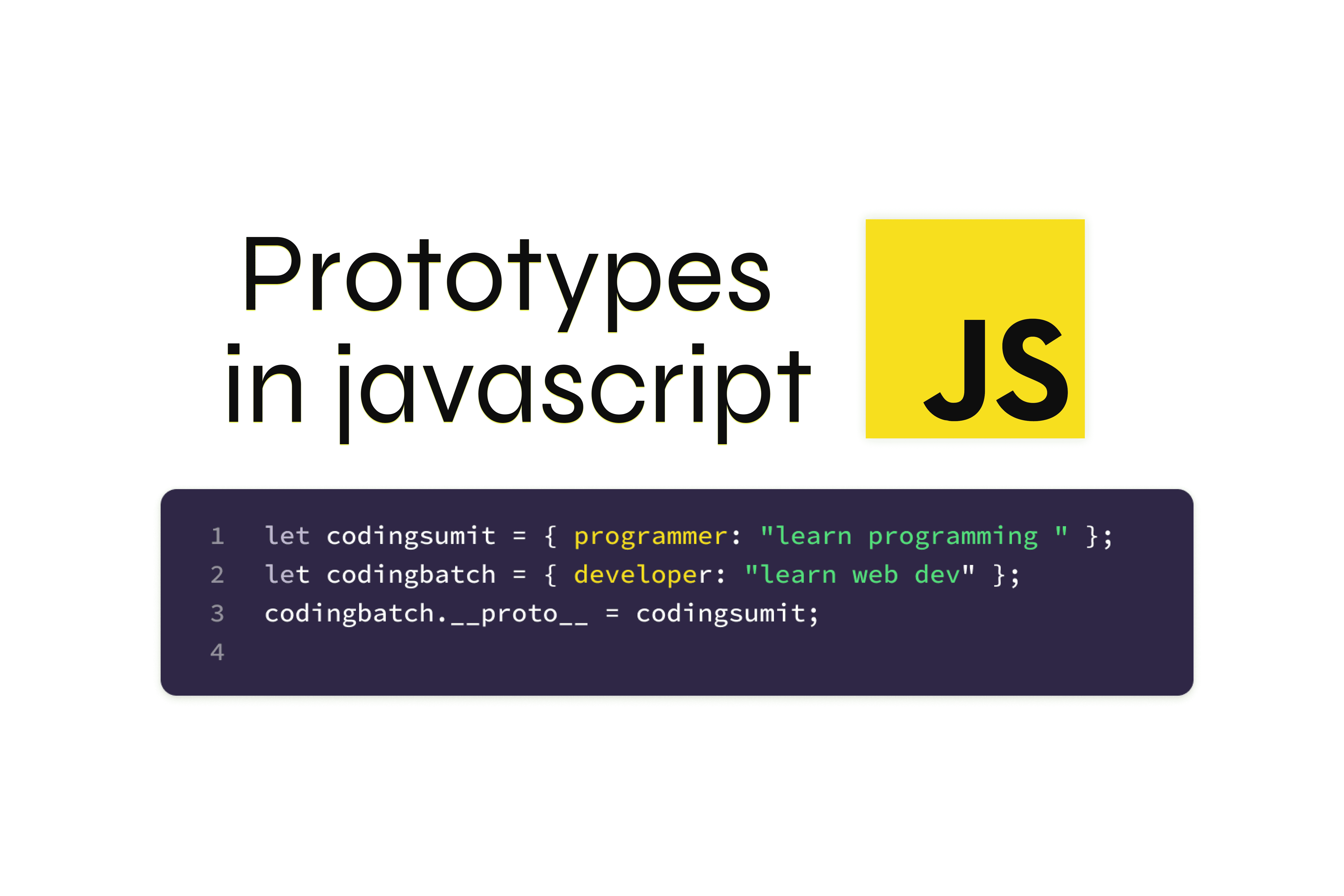
In this tutorial we'll learn about prototypes in javascript and their methods and features in depth with examples
In JavaScript, objects have a special hidden property known as Prototype
which depicts either null or references another object. Now, that object is called a prototype. In this advanced JavaScript Tutorial, we will discuss two important features of prototype
- Prototypal Inheritance
- Prototype methods
🔗Prototypal Inheritance
When we read a property from object, and if it's missing javascript automatically takes it from the prototype this is called Prototypal Inheritance
Let's learn Prototypal Inheritance
with a one simple example for better understanding
Let’s see we have a two different object codingbatch and codingsumit where first object contains programmer property and second contains developer property
jslet codingsumit = { programmer: "learn programming " };let codingbatch = { developer: "learn web dev" };
lets set codingbatch object to codingsumit object by using proto in programming this is called Prototypal inheritance
jscodingbatch.__proto__ = codingsumit; // sets codingbatch to codingsumit.
Now if we read a property from codingbatch, and if property missing, JavaScript will automatically take it from codingsumit.
we can find both properties developer
and programmer
in codingbatch now:
jsconsole.log(codingbatch.programmer); // learn programmingconsole.log(codingbatch.developer); // learn web dev
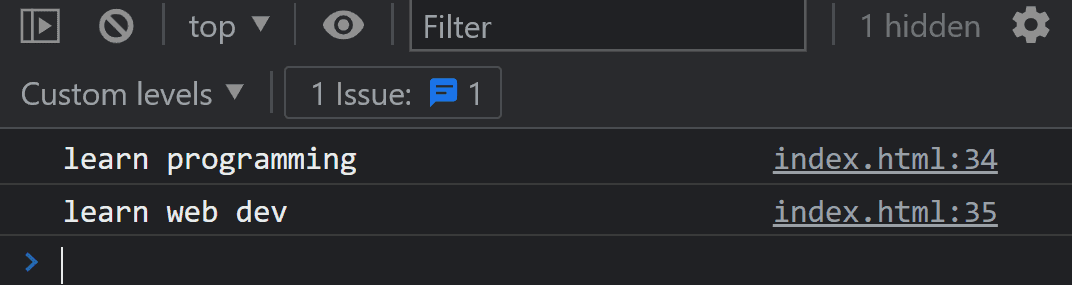
🔗Prototypal method
The __proto__
is considered outdated and somewhat deprecated (in browser only part of the JavaScript standard).
The modern methods are:
Object.create(proto, [descriptors])
Object.getPrototypeOf(obj)
Object.setPrototypeOf(obj, proto)
Object.create(proto, [descriptors])
:- creates an empty object with given proto as[[Prototype]]
and optional property descriptors.
jslet codingbatch = { programmer: "learn programming" };// create a new object with codingbatch as a prototypelet codingsumit = Object.create(codingbatch);console.log(codingsumit.programmer); // learn programming
![ProtoObject.create(proto, [descriptors])](/static/e939ec66ee83250e19ec2ccf5b18dcb6/17446/1.png)
Object.getPrototypeOf(obj)
: – returns the [[Prototype]] of obj.
jslet codingbatch = { programmer: "learn programming" };let codingsumit = Object.create(codingbatch);console.log(Object.getPrototypeOf(codingsumit));
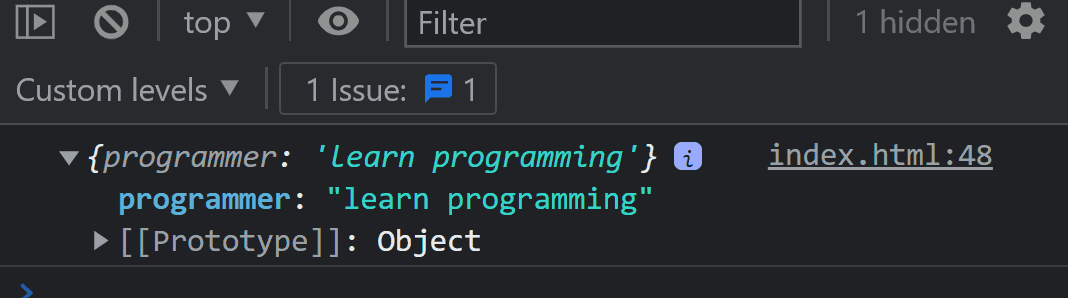
Object.setPrototypeOf(obj, proto)
: – sets the [[Prototype]] of obj to proto.
jslet codingbatch = { programmer: "learn programming" };let codingsumit = Object.create(codingbatch);Object.setPrototypeOf(codingsumit, {programmer: "javascript programming",});console.log(codingsumit.programmer);