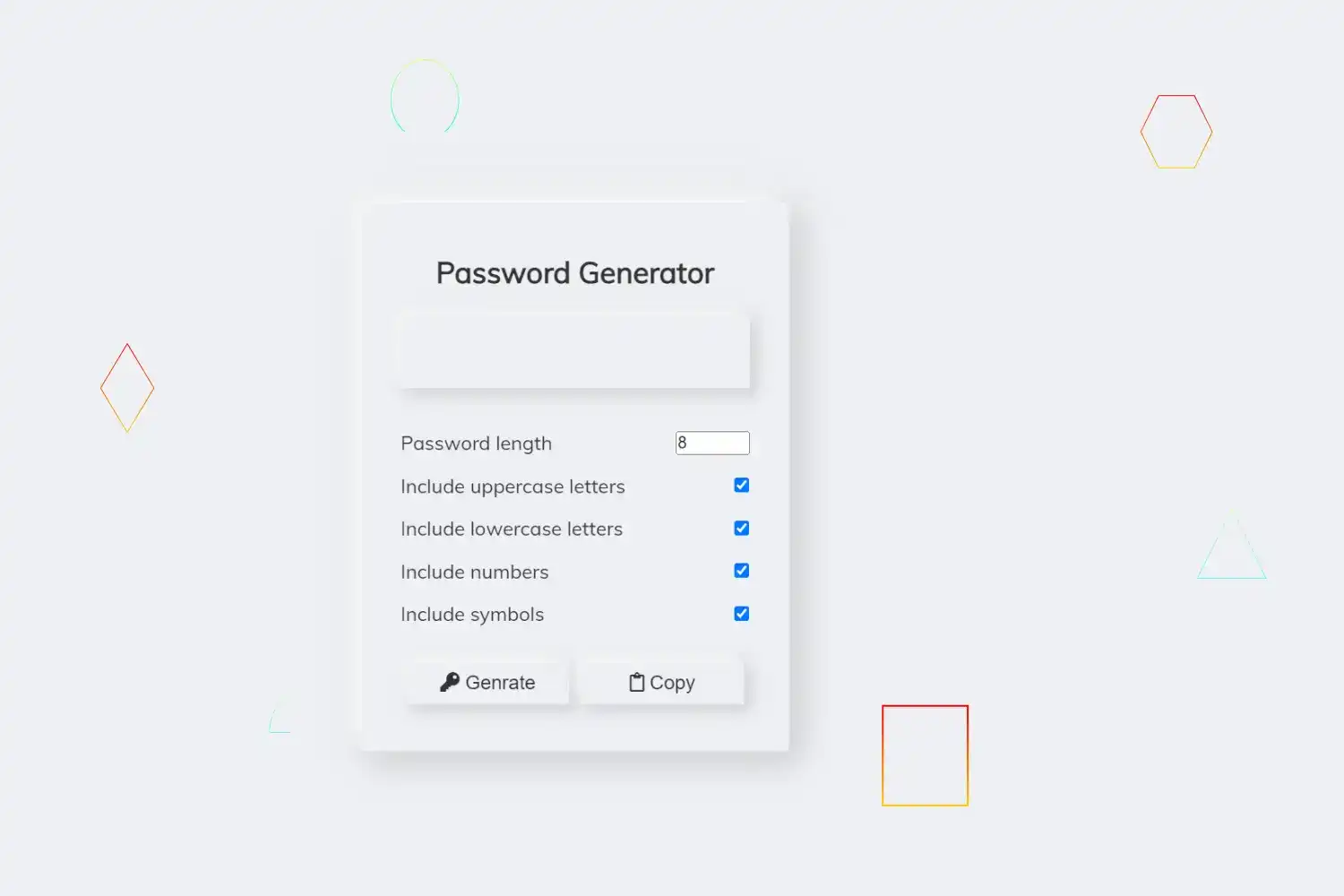
In this tutorial we’re going to learn how to create a amazing password generator using html css and javascript with the dark light mode functionality
🔗Prerequisites
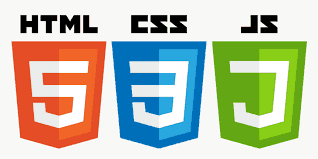
🔗Get started ✌
The password generator we’ll build in this tutorial will be pretty cool. a user can generate a password by clicking generate button between 1 to 20 characters length including lowercase uppercase string , number , symbol also copy a generated password
I’ll explain how to build each feature, but you must follow along by typing the code and running it on your end to get the most out of this tutorial
Click here to get starter files
🔗First let’s create the skeleton of the ui
html<div class="container"><h2>Password Generator</h2><div class="result-container"><textarea id="PasswordResult"></textarea></div><div class="settings"><div class="setting"><label>Password length</label><input type="number" id="Passwordlength" min="4" max="20" value="8" /></div><div class="setting"><label>Include uppercase letters</label><input type="checkbox" id="uppercase" checked /></div><div class="setting"><label>Include lowercase letters</label><input type="checkbox" id="lowercase" checked /></div><div class="setting"><label>Include numbers</label><input type="checkbox" id="numbers" checked /></div><div class="setting"><label>Include symbols</label><input type="checkbox" id="symbols" checked /></div></div><div class="buttons"><button class="btn btn-large" id="generateBtn"><i class="fas fa-key"></i> Generate</button><button class="btn" id="clipboardBtn"><i class="far fa-clipboard"></i> Copy</button></div></div>
Output of above code 👇
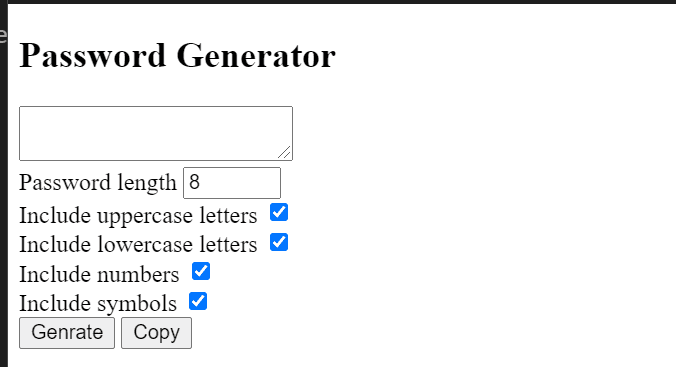
🔗Let’s add styling into ui 🎨
Creating css variables for colors, typography, visuals etc .
css:root {--bg-color: #eef0f4;--color: #333;--togglebg: #333;--roundcolor: #eef0f4;--toggleslider: #111;--togglesliderColor: #111;--filter1: drop-shadow(-8px -8px 12px #ffffff) drop-shadow(8px 8px 12px #c3c5c8);--filter2: drop-shadow(-4px -4px 6px #ffffff) drop-shadow(4px 4px 6px #c3c5c8);--filter3: drop-shadow(-2px -2px 3px #ffffff) drop-shadow(2px 2px 3px #c3c5c8);}
Basic styling
css/* basic styling */* {box-sizing: border-box;margin: 0;padding: 0;}/* referencing css bg color and color variable in body */body {/* visuals */background-color: var(--bg-color);color: var(--color);/* typography */font-family: "Muli", sans-serif;margin: 10%;}p {/* typography */margin: 5px 0;}h2 {/* box model */margin: 10px 0 20px;text-align: center;}
Styling main container , referencing --bg-color,--filter1 css variables
css.container {/* box model */margin: auto;padding: 20px;width: 350px;max-width: 100%;/* visuals */background: var(--bg-color);border: 12px solid var(--bg-color);filter: var(--filter1);}
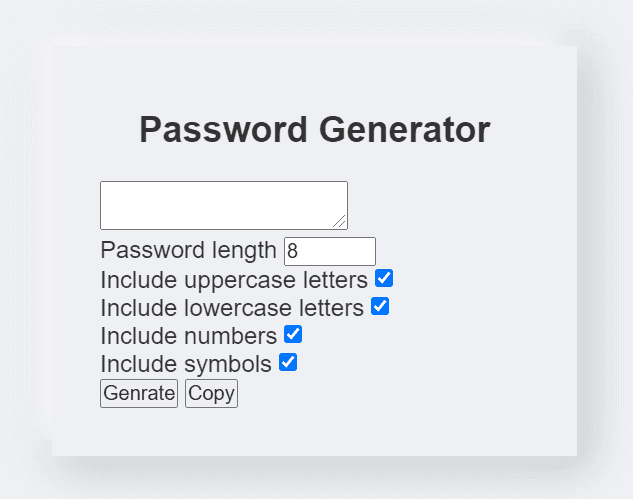
Styling .result-container , referencing --bg-color, --filter2 css var
css.result-container {/* visuals */background-color: var(--bg-color);border: 12px solid var(--bg-color);filter: var(--filter2);/* box model */display: flex;justify-content: flex-start;align-items: center;height: 60px;width: 100%;margin-bottom: 35px;/* positioning */position: relative;/* typography */font-size: 18px;letter-spacing: 1px;}
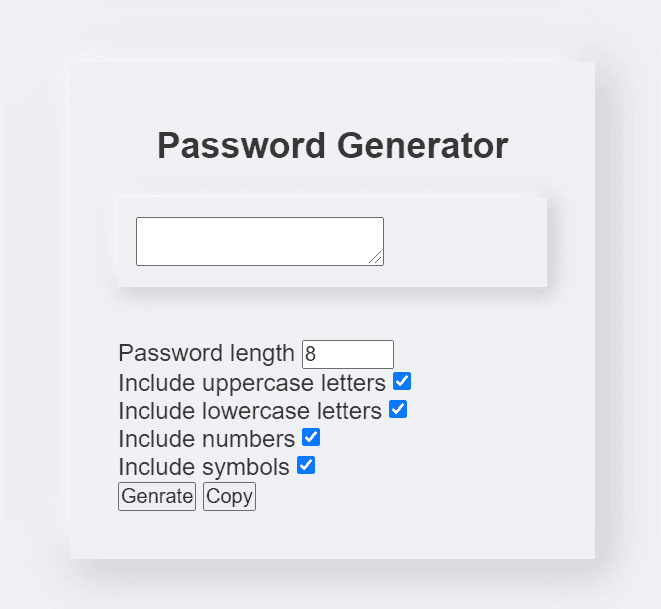
Styling textarea , referencing --color css var
csstextarea {background: none;border: none;color: var(--color);font-size: 20px;margin-top: auto;outline: none;resize: none;}
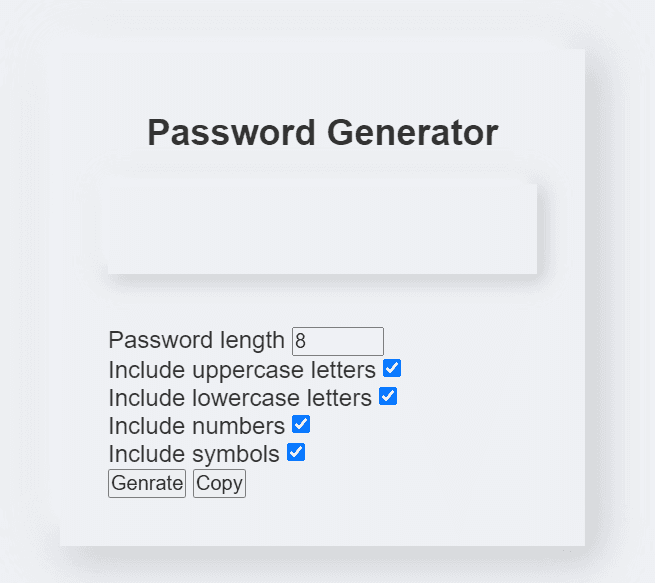
Setting align the flex items space between
css.setting {display: flex;justify-content: space-between;align-items: center;margin: 15px 0;}
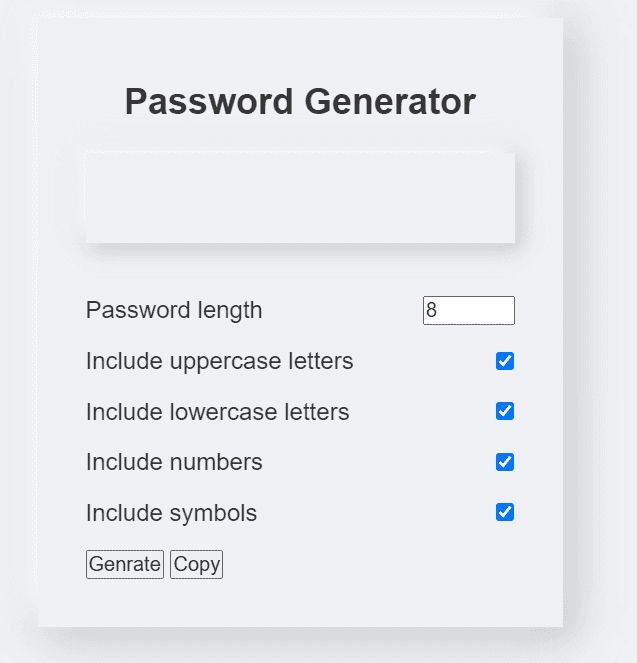
Styling buttons
css.btn {width: 50%;border: none;color: var(--color);cursor: pointer;font-size: 16px;padding: 8px 12px;margin: 14px 5px 7px 5px;background-color: var(--bg-color);filter: var(--filter2);}.btn:hover {filter: var(--filter3);transition: 0.3s ease-in-out;}
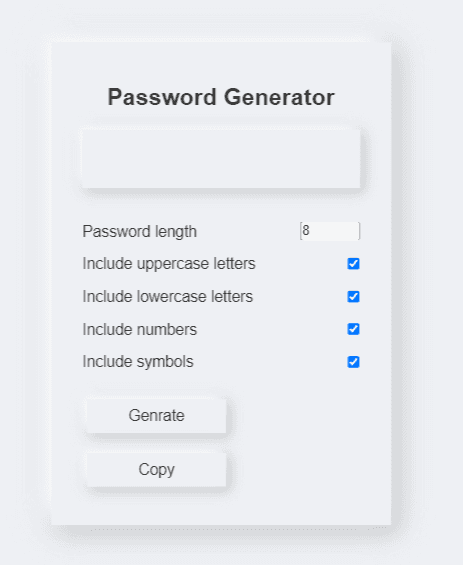
Setting flex to buttons for displaying buttons in a row
css.buttons {display: flex;}
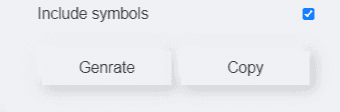
See the complete code at the end of this step
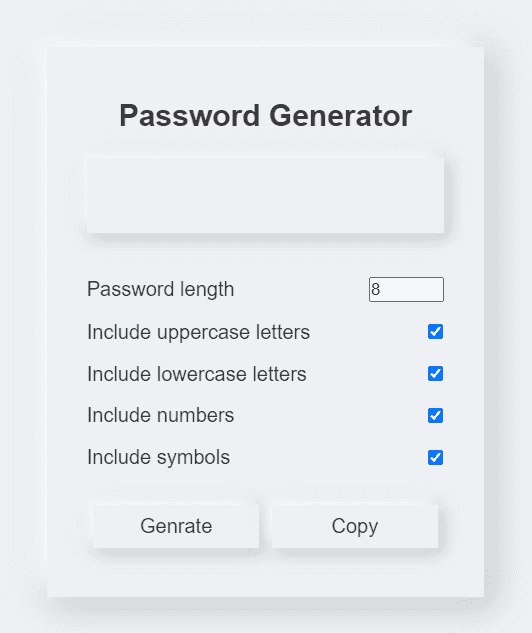
🔗Let’s start building feature for generate random password and copy password
Function for generating random lowercase, uppercase, number, symbol
1. Creating function for generating random lowercase letter
For generating lowercase letters we can use String.fromCharCode() method With this method we’ll get the certain character based on their code
For example 97 is a lowercase “a” see in below image
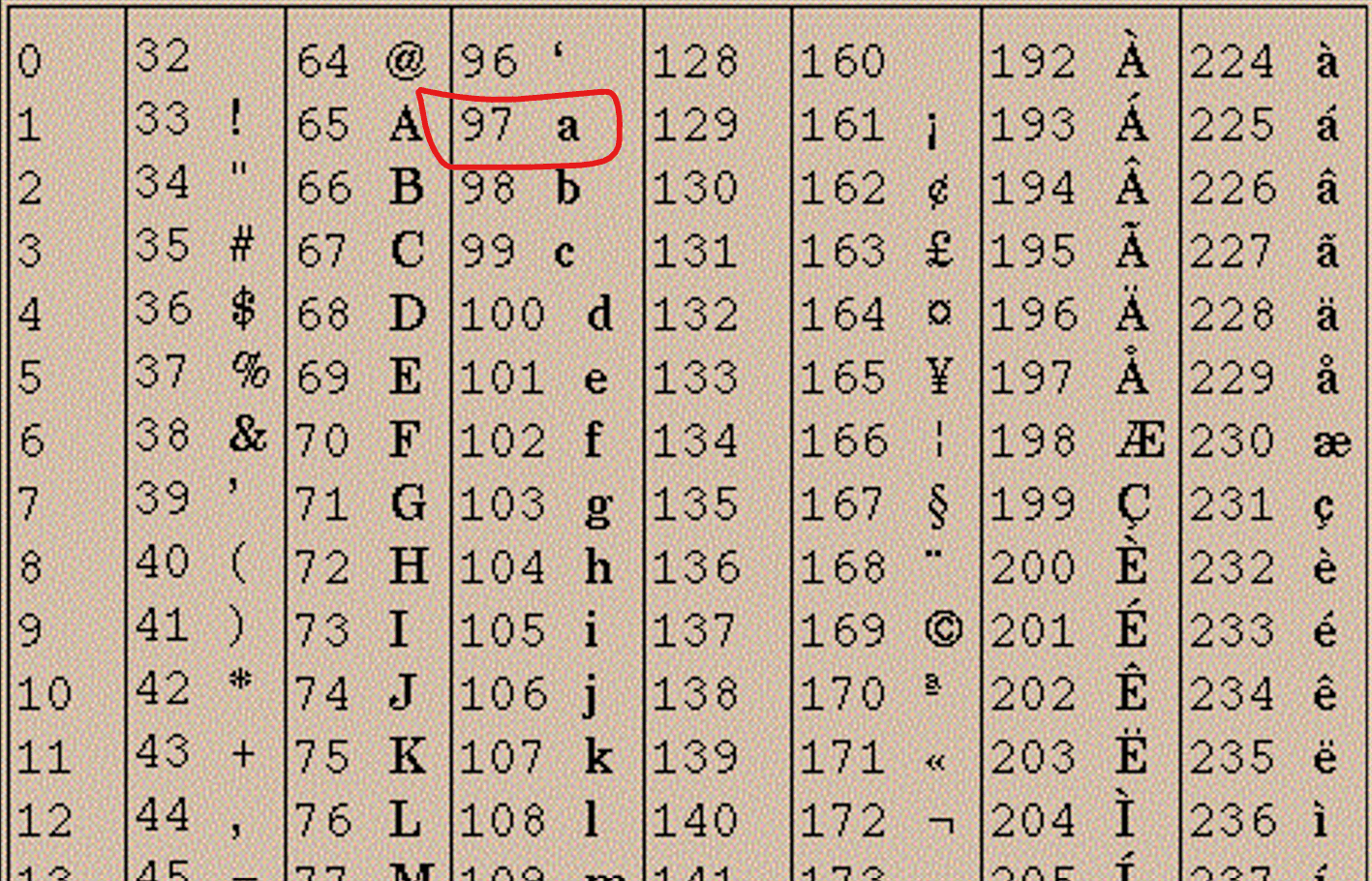
As you can see below in console we’re getting “a” by using String.fromCharCode(97)
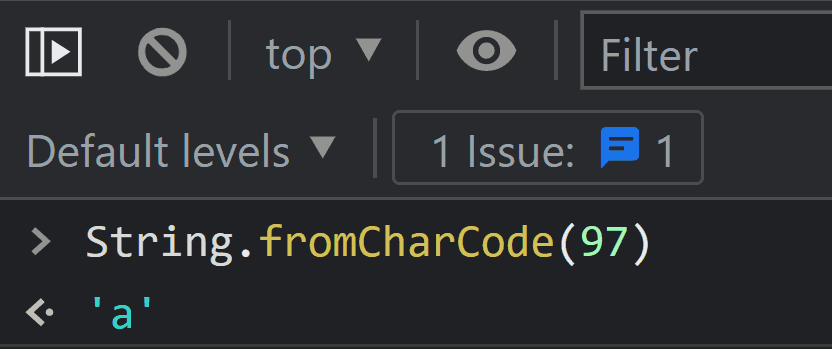
as you can see uppercase “A” is 65 , Number 1 is 49 in the image below
Charcode image 👇
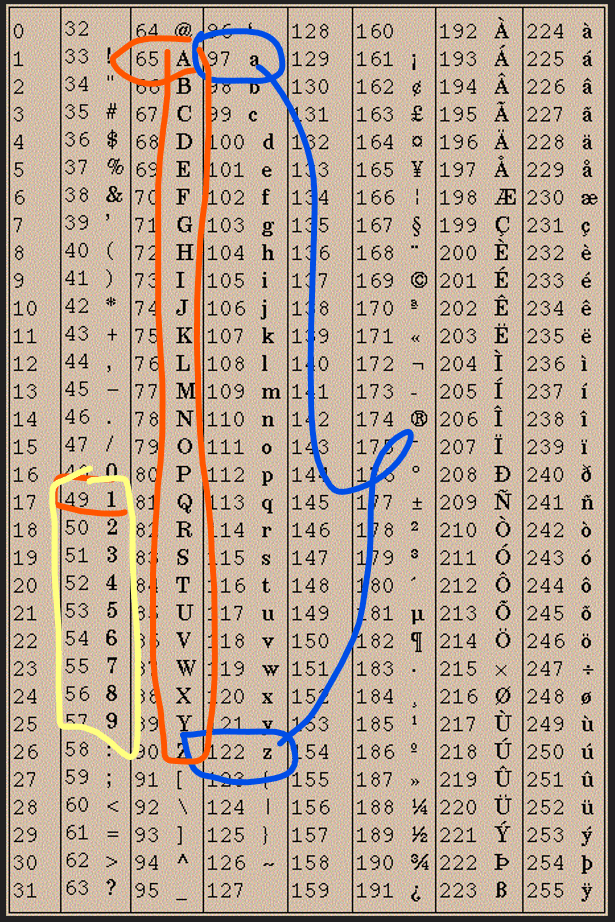
So keep this in mind this charcode set when we are dealing with this
You can find full list of charcode here download or open this in a new tab
For generating random lowercase, uppercase letters, number , symbols we can use Math.floor with Math.random method let’s first understand how actually math.floor and Math.random method works !
We want random integers for that we can use Math.random() method to returns a random integers
jsconsole.log(Math.random() * 26);
as you can see that we're getting a random floating number between 0 to 26 like 18.8 , 10.23 , 6.09… etc .. 👇
But we don't want floating number we want whole number so in this case we can use Math.floor method, Math.random() with Math.floor() can be used to return a random whole integer
Below code will return random whole number (from limit up to 26) to (starting from 1)
jsconsole.log(Math.floor(Math.random() * 26 + 1));
Now we understood what is actually Math.floor and Math.random method let’s implement both to generate a lowercase letter from charcode which is 97 to 122 see on image , for that we have to set the limit to upto 26 random integers between 1 to 26 to 97 because of we have 26 letters in alphabet
jsconsole.log(String.fromCharCode(Math.floor(Math.random() * 26) + 97));
Above code return random lowercase letters based charcode between 97 to 122
Now just return the above expression in getRandomLower function
jsfunction getRandomLower() {return String.fromCharCode(Math.floor(Math.random() * 26) + 97);}console.log(getRandomLower());
Yeah we have successfully created a getRandomLower function 🤪 🎉
Now let’s go further and create function for uppercase, number, symbols by using too by same methods 🙌
2. Creating function for generating random uppercase letter
Similarly we can do for generating a Random Uppercase letter now if we look at the Char code chart the uppercase “A” is start at 65 so what we want to do there's still 26 letters right there so we're gonna just add 65 and we're gonna get random uppercase letter between 65 to 90 charcode
jsfunction getRandomUpper() {return String.fromCharCode(Math.floor(Math.random() * 26) + 65);}console.log(getRandomUpper());
Above code return random uppercase letters based on charcode between 65 to 90
3. Creating function for generating random numbers
Similarly we can do for generating a Random numbers as we can see in the chart a number 0 is start at 48 so we're gonna just add 48 to upto total number of numbers 48 to 57 = 10 random integer number between 0 and 9 to 48 using charcode
jsfunction getRandomNumber() {return String.fromCharCode(Math.floor(Math.random() * 10) + 48);}console.log(getRandomNumber());console.log(typeof getRandomNumber());
Above code return random numbers based on charcode between 48 to 57
but as you can see above ☝️ we're getting number values in a string to convert it to number we can use Unary plus (+)
jsfunction getRandomNumber() {return +String.fromCharCode(Math.floor(Math.random() * 10) + 48);}console.log(getRandomUpper());
Now you can see 👇 we’re getting number values as a typeof number 🤟
4. Creating function for generating random symbols
Similarly we can generate random symbols as we can see in the chart a symbol ‘!’ is start at 33 so we're gonna just add 33 to upto total number of symbols 33 to 47 = 15
jsfunction getRandomSymbol() {return String.fromCharCode(Math.floor(Math.random() * 15) + 33);}console.log(getRandomUpper());
Above code return random symbols based on charcode between 33 to 47
Congratulation we have successfully created a function for generating random lowercase, uppercase letters, number, symbol
See the complete code at the end of this step
Putting all generator function into randomFun object
For now let’s just put all function into a randomFun object we'll use it for generating random password later in this tutorial
jsconst randomFunc = {// keys : valuelower: getRandomLower,upper: getRandomUpper,number: getRandomNumber,symbol: getRandomSymbol,};
Let’s add a click event listener to generate button
get button dom element by using getElementById then add click event listener to generate button
jsgenerate.addEventListener("click", () => { }
after click button we want a value of password length and value of checked unchecked lowercase ,uppercase, number, symbol options
- We can get the value of password length by using .value getter method
- We can get the value of option checked or unchecked by using the .checked getter method. This method will return true if the checkboxes are checked Otherwise, it’ll return false.
jsgenerate.addEventListener("click", () => {const length = document.getElementById("Passwordlength").value;const hasUpper = document.getElementById("uppercase").checked;const hasLower = document.getElementById("lowercase").checked;const hasNumber = document.getElementById("numbers").checked;const hasSymbol = document.getElementById("symbols").checked;}
For now if we console.log(hasUpper, hasLower, hasNumber, hasSymbol, length); On a button click event we’ll get the value of length , checked unchecked options
jsconsole.log(hasUpper, hasLower, hasNumber, hasSymbol, length);
See the output in console log 👇
We have to pass these values as an argument in our main function which is generatePassword function we'll create that function later in this tutorial because after clicking generate button we want to call our main function which is generatePassword To get the value of generated password
jsgeneratePassword(hasLower, hasUpper, hasNumber, hasSymbol, length);
Let’s create our main function generatePassword for generating a random password
We have to create generatePassword function with the four parameters because we have previously assigned the generatePassword function with the fourarguments in generate button click event listener
In generatePassword function we’ll gonna
- Init generatedPassword empty string ( for storing our password )
- Counting a number of checked values ( we’ll use this in the for loop later in this tutorial )
- Filter out unchecked values ( we’ll use this in forEach later in this tutorial )
- Creating a loop for calling generator function for each type
- Let’s start with Init generatedPassword empty string
jsfunction generatePassword(lower, upper, number, symbol, length) {let generatedPassword = "";}
- Counting a number of checked values if we checked 3 options it's return 3 , if we checked 2 options it's return 2
jsfunction generatePassword(lower, upper, number, symbol, length) {let generatedPassword = "";const typesCount = lower + upper + number + symbol;}
👇 For now if we console.log(typesCount); we’ll get the count value of checked options
- Filter out unchecked values we want to generate random password based on only checked value for that we have to filter out unchecked value we can archive that by using array filter method to achieve that we have to first convert it into array for that we have to pass all parameters as an array object
jsconst typesArr = [{ lower }, { upper }, { number }, { symbol }];
👇 If we console.log(typesArr)
it will return value of checked , unchecked which is true of false
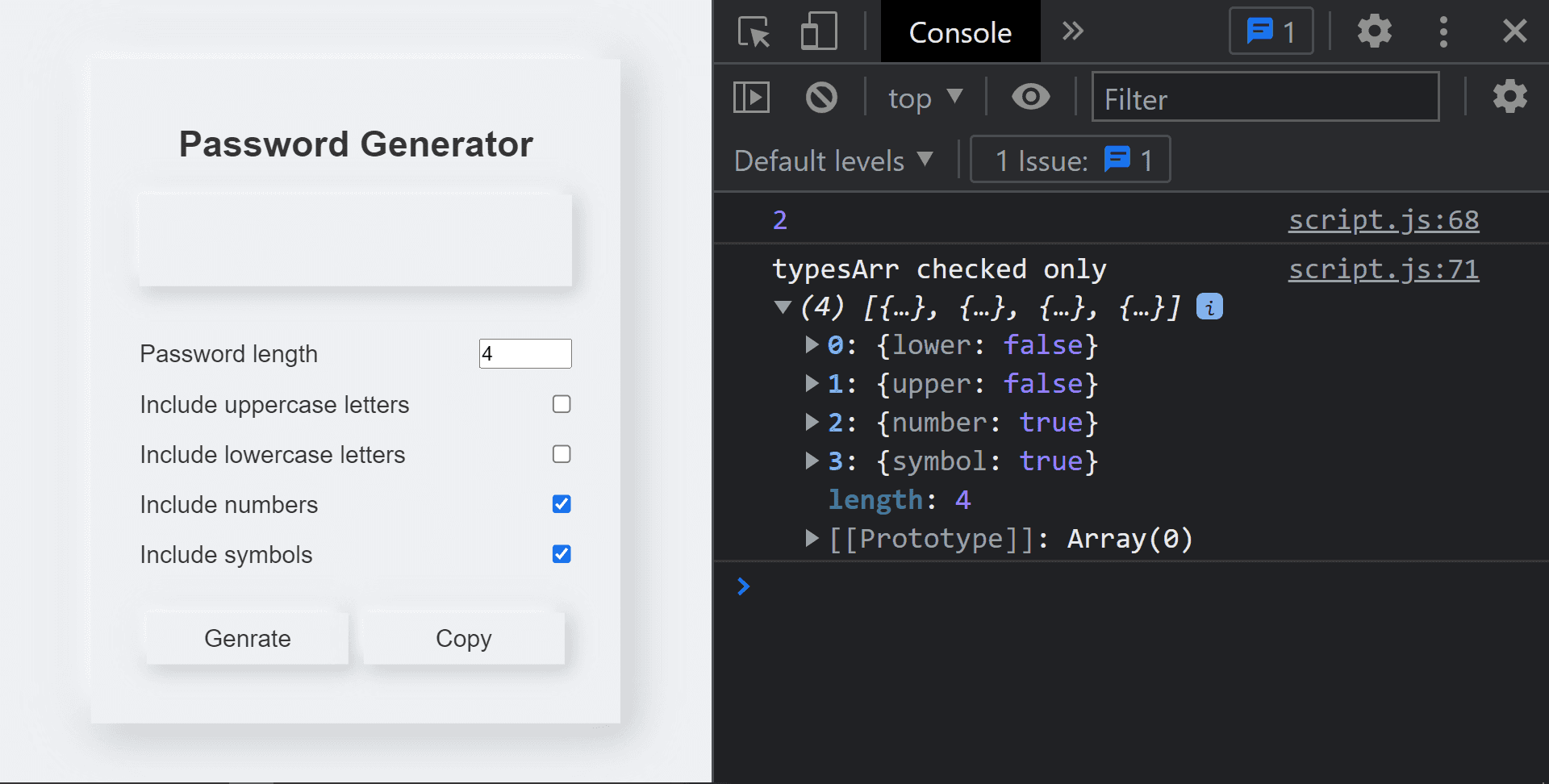
We don’t want value of unchecked we have to filter out unchecked false values to archive that we can use filter array method Now let’s use filter method
jsconst typesArr = [{ lower }, { upper }, { number }, { symbol }].filter((item) => Object.values(item)[0]);
👇 Now If we console.log(typesArr)
it will return value of only checked which is true
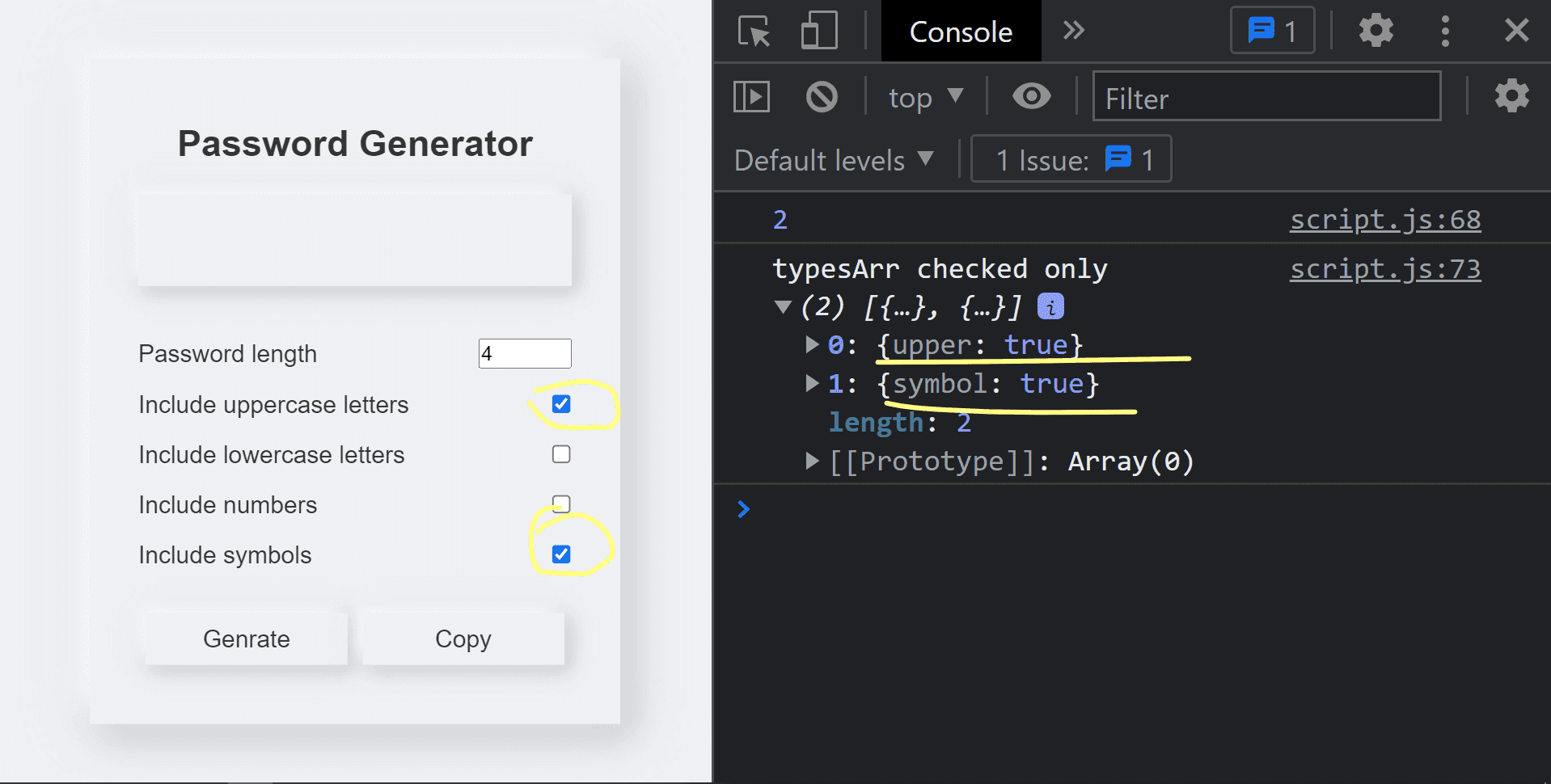
4. Creating a loop for calling generator function for each type
Now we have to call a our generate function for each type of typesArr what we created
at the beginning using String.fromCharCode()
method for that we can use forEach method
eg : when we have only lower option checked then we want to call getRandomLower function only for lowercase letters
jstypesArr.forEach((type) => {const funcName = Object.keys(type)[0];generatedPassword += randomFunc[funcName]();// For testing purposeconsole.log(funcName, generatedPassword);console.log("generatedPassword =", generatedPassword);});
In above code we : -
assign a key value of typesArr object forEach type of typesArr to funcName using Object.keys method for getting the value of Object.keys for each type of typesArr
assign a generatedPassword = generatedPassword + randomFun(funName()) for calling funcName in randomFun object to access the keys value of randomFunc object
So now if we run the above code we’ll get the generated password 👇
As you can see above ☝️ we're getting the random password but there we have a oneproblem we set the length of password by default 4 in html but when we unchecked a other options we're getting a random password based on typeCount value to solve that
We have to create a loop for calling generator functions what we created at the beginning using String.fromCharCode() method , for each type based on password length value . For that we can use for loop just simply create a for loop and paste the above forEach expression within a for loop block
jsfor (let i = 0; i < length; i += typesCount) {typesArr.forEach((type) => {const funcName = Object.keys(type)[0];generatedPassword += randomFunc[funcName]();// For testing purposeconsole.log(funcName, generatedPassword);console.log("generatedPassword =", generatedPassword);});}
Now we’re getting the password based on password length value 👇
But here we have another problem when we updated and set the value of password length to above or below 4, for example length = 3 we still getting the 4 characters password the reason for that is because in for loop we're incrementing by whatever the typesCount
to solve this we have to slice password from 0 to length using slice method which mean if we set length = 1 it well slice password from 0 to 1 now if we unchecked options we getting password based on length of password
Slicing password
jsconst finalPassword = generatedPassword.slice(0, length);// for testing purposeconsole.log(finalPassword);
Now we get proper random password without any problem 👇
See the complete code at the end of this step
Assigning password into result
But we want random password in textarea as a result after click generate button
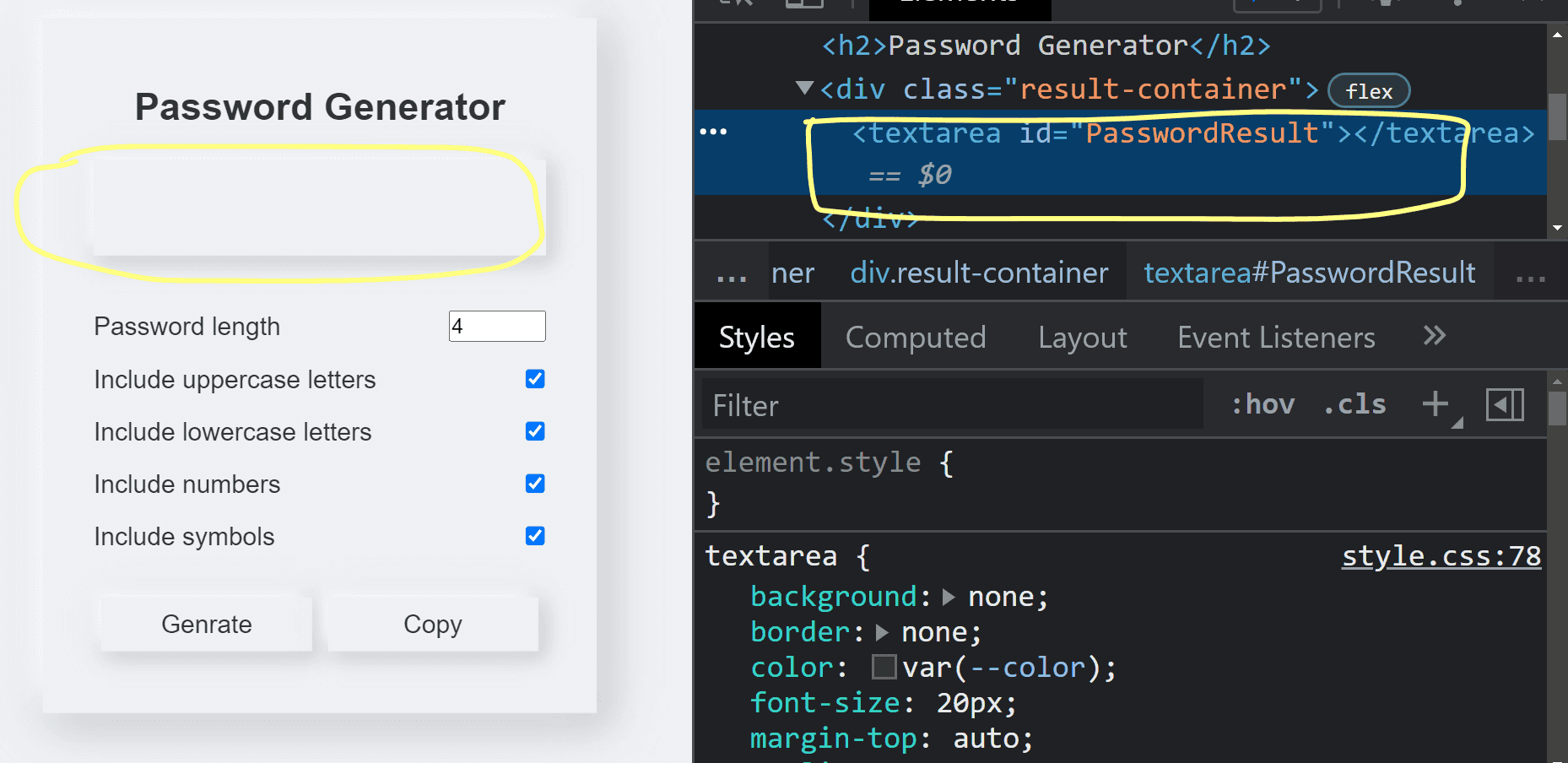
For that we have to select dom element textarea using getElementById PasswordResult in a generate button click event listener block and call our generatePasssword function in element.innerText
js// getting dom element textarea by id PasswordResult and assigning into result variableconst result = document.getElementById("PasswordResult");
As previously we called our generatePasssword() function with four argument in click event listener we have to just cut and paste into result.innerText
js// getting dom element textarea by id PasswordResult and assigning into result variableconst result = document.getElementById("PasswordResult");result.innerText = generatePassword(hasLower,hasUpper,hasNumber,hasSymbol,length);
Now if we click on generate button we getting undefined the reason is that we haven’t returned our finalPassword after slicing generatedPassword , we console logged our final password for getting the final password in console.log 👇
To get final passwordin result we have to just return finalPassword after slicing
jsconst finalPassword = generatedPassword.slice(0, length);// for testing purpose// console.log(finalPassword);return finalPassword;
Now if we click on generate button we got random password as expected 👇
Let’s create copy generated password feature
We can create copy generated password feature easily by using execute command
- We have to get the copy button dom element using getElementById
- Add click event listener to button
- Apply exe command for copy text by selecting textarea text with id
jslet button = document.getElementById("clipboardBtn");button.addEventListener("click", (e) => {e.preventDefault();document.execCommand("copy",false,document.getElementById("PasswordResult").select());});
See below 👇
Congratulation we have successfully created password generator app 🥳