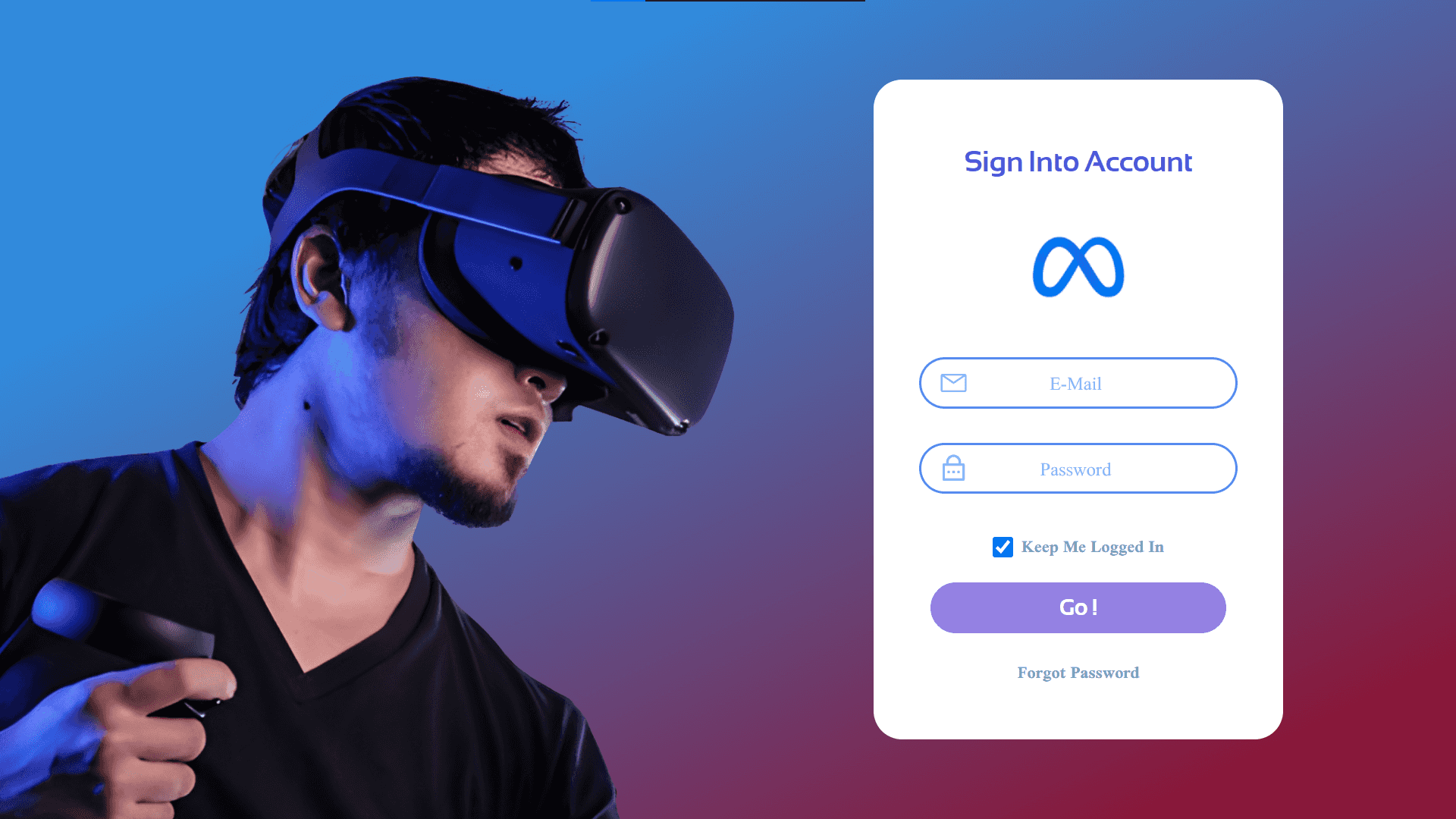
In this tutorial we’re going to learn how to create a responsive login page using html css and javascript
Click here to get starter files
🔗First let’s create the main container
( Define main container and assign svg gradient background with img )
Markup of main container
html<div class="main-container"></div>
Styling main-container
( setting background image and style for background and main-contianer )
css/* basic styling */* {margin: 0;padding: 0;box-sizing: border-box;}/* styling .main-container */.main-container {background-image: url(images/bg.svg);background-size: cover;height: 100vh;}
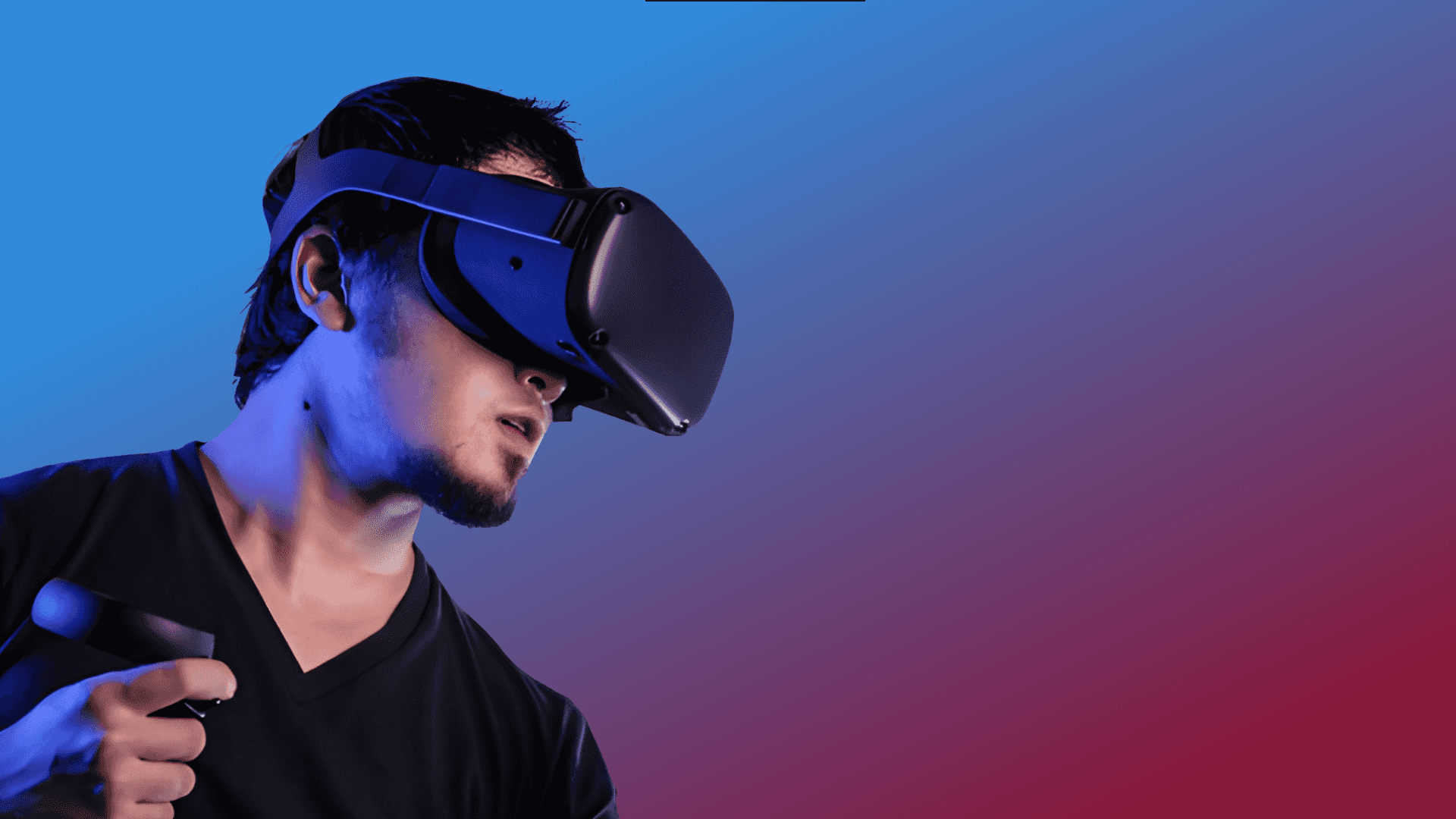
🔗Create login form in right side
( We want login form in right side of main-container for that add div inside main-container )
Markup of right side div container ( update html as follow )
html<div class="main-container"><div class="right"></div></div>
Styling right side div
css.right {position: absolute;width: 360px;left: 60%;height: 580px;top: calc(50% - 580px / 2);background: #ffffff;border-radius: 25px;text-align: center;}
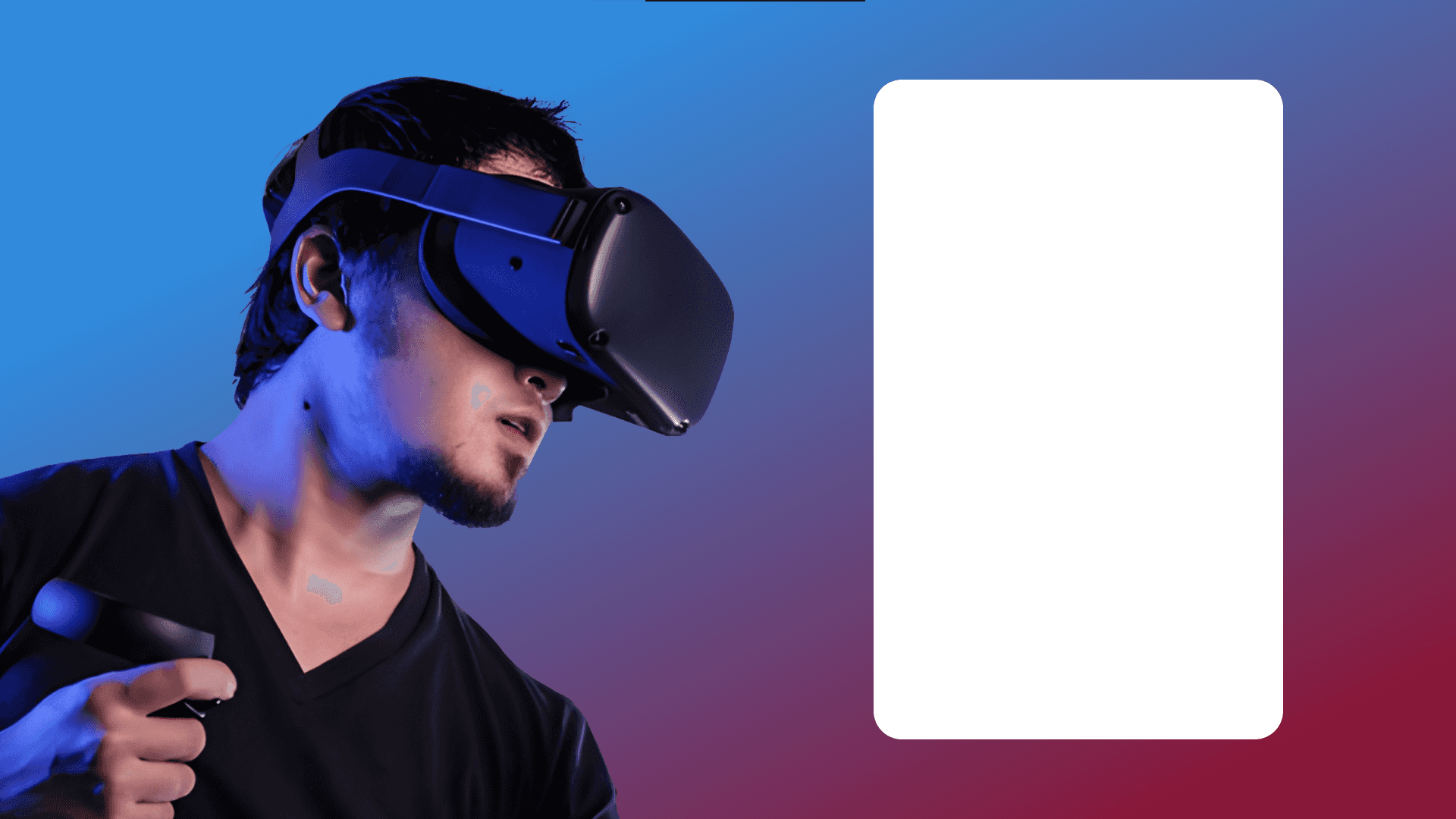
🔗Let's create title of form and logo
Markup of title and logo ( update html as follow )
html<div class="main-container"><div class="right"><h1 class="title">Sign Into Account</h1><img class="logo" src="images/logo.png" alt="" /></div></div>
Styling title of form and logo
css.title {font-family: Sansation;font-style: normal;font-weight: bold;font-size: 25px;line-height: 145px;align-items: center;font-family: "Sansation", sans-serif;color: #5754dd;}.logo {width: 120px;height: 120px;margin-top: -40px;}
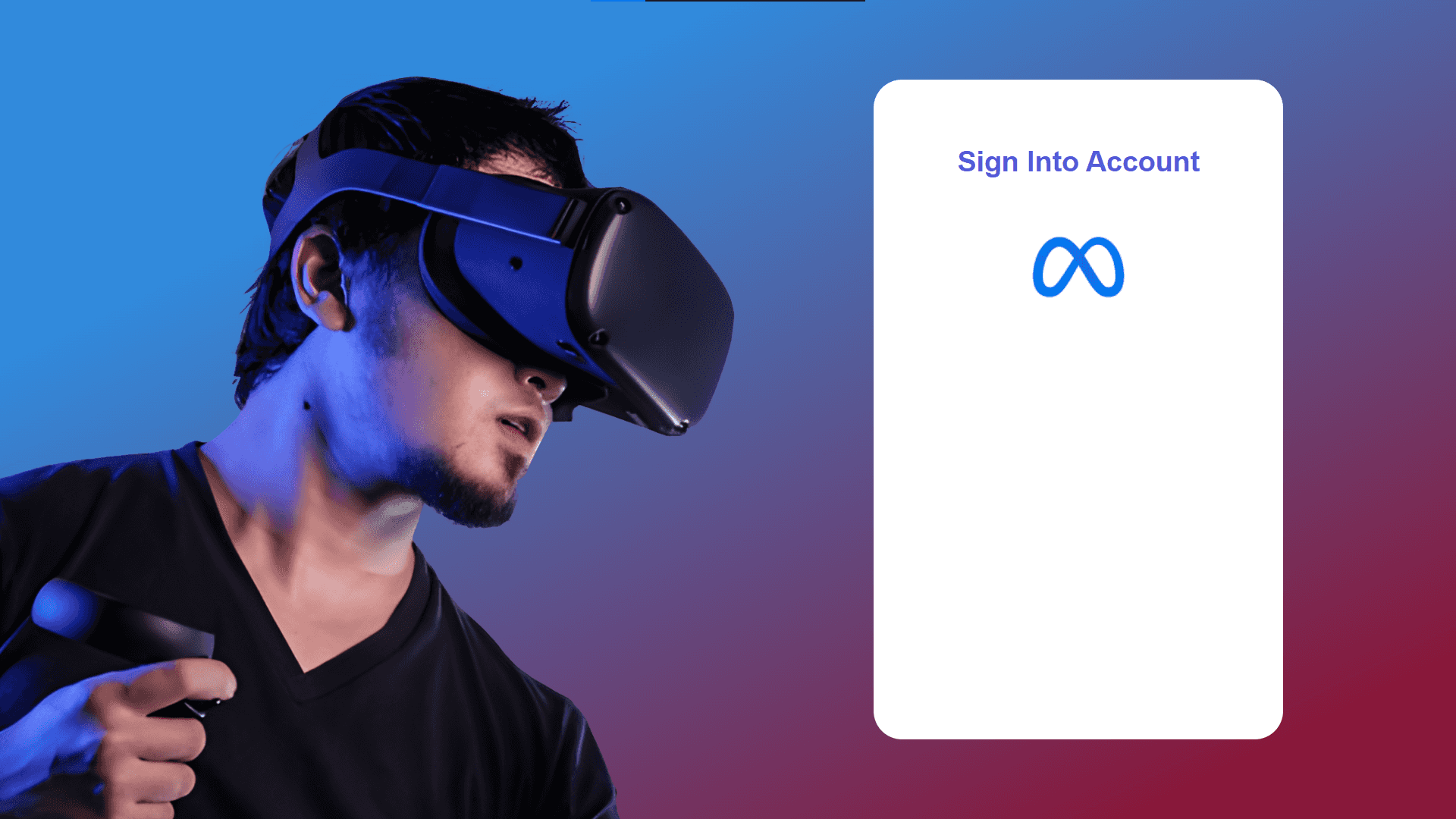
🔗Let's create our main form container where we'll create inputs field and submit button
Markup of form container and input fields
html<div class="form-container"><div class="username"><img class="icon email-icon" src="icons/email.svg" alt="" /><input type="email" class="user-input" placeholder="E-Mail" /></div><div class="password"><img class="icon password-icon" src="icons/password lock.svg" alt="" /><input type="password" class="pass-input" placeholder="Password" /></div></div>
Styling username and password input fields
cssinput[type="email"],input[type="password"] {border: none;outline: none;background: none;font-size: 20px;color: #85b6ff;padding: 20px 10px 20px 5px;border: 2px solid #568aee;width: 280px;height: 45px;margin: 15px;border-radius: 25px;box-sizing: border-box;text-align: center;overflow: hidden;font-family: "Scheherazade New", serif;}
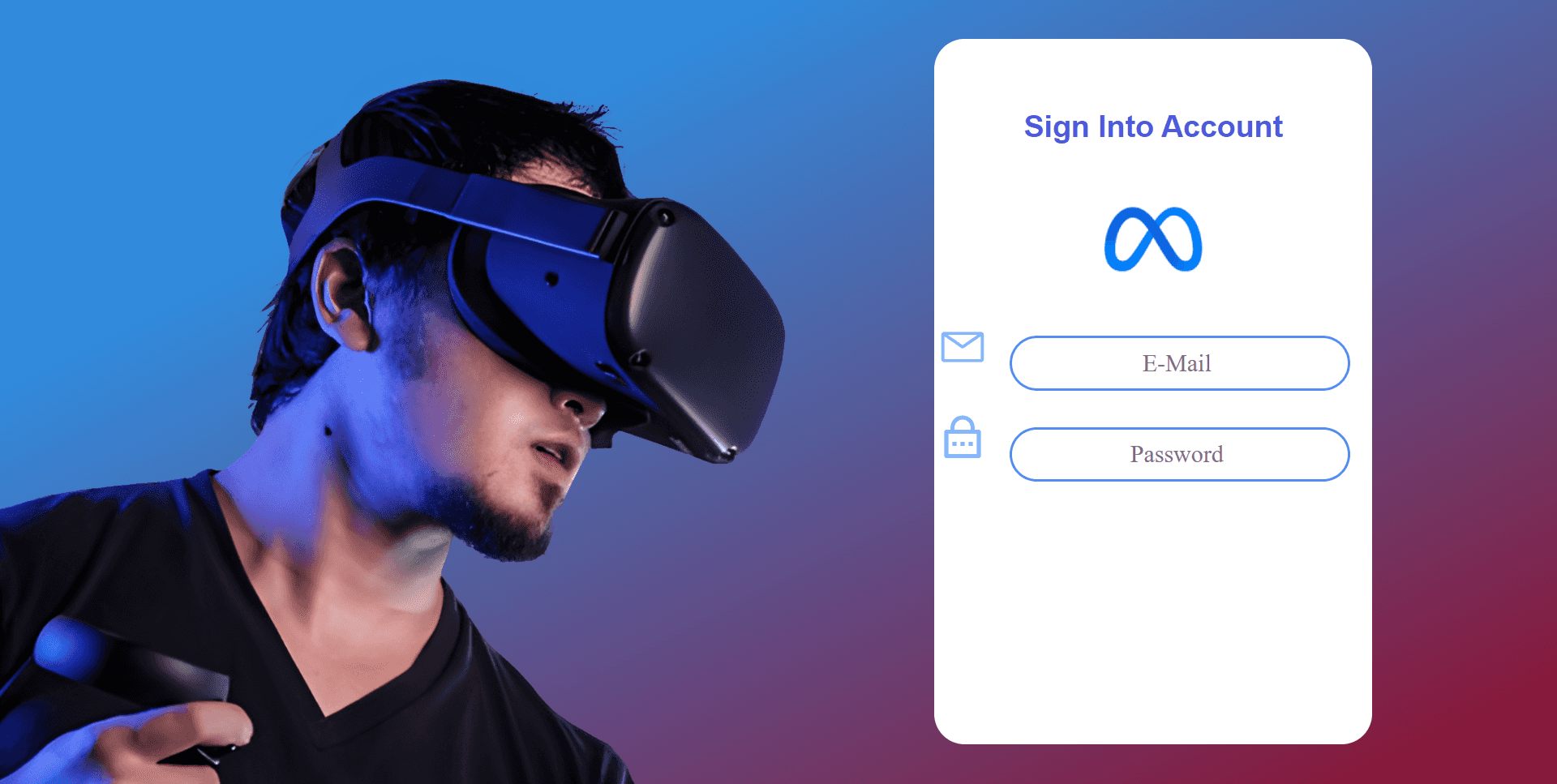
Style to change the color of placeholder text
( we can add custom styling to placeholder using ::placeholder selector )
css::placeholder {color: #85b6ff;font-size: 18px;}
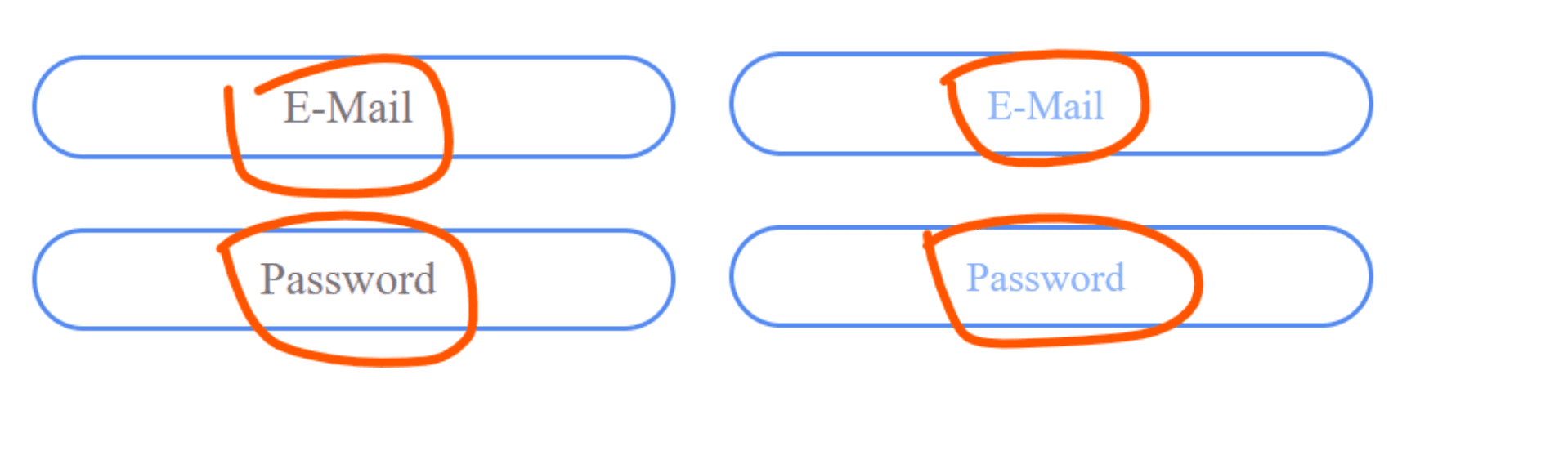
Style icons to place icon inside input field
css.icon {position: absolute;padding: 24px 32px;height: 75px;width: 90px;}
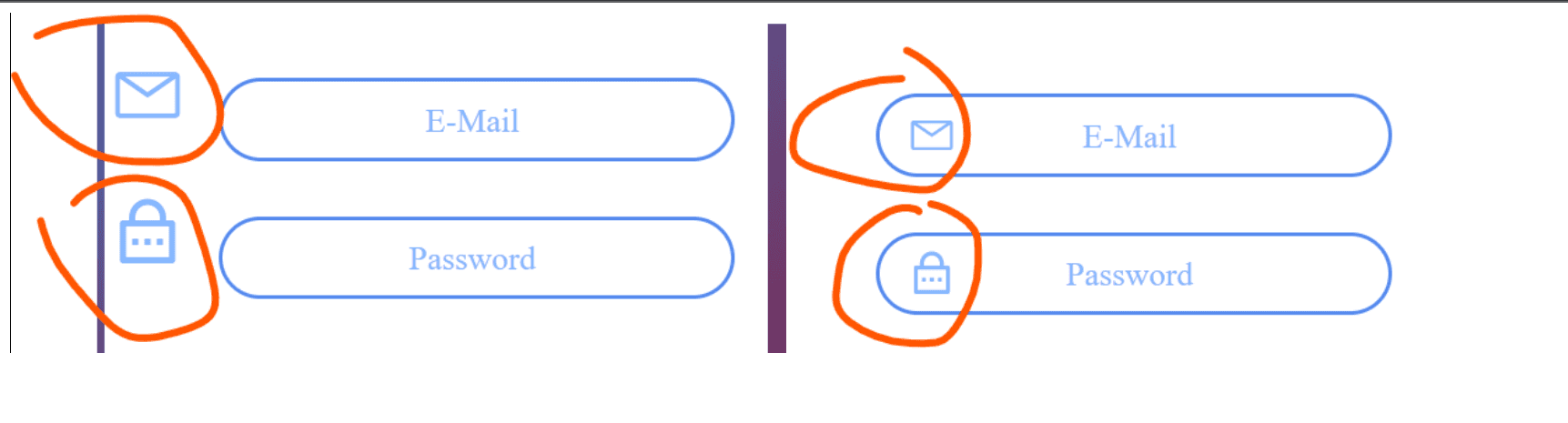
🔗Let’s create keep me checkbox , submit button and forgot link
Markup of keep me text with checkbox , submit form button and forgot password link
html<div class="keepme"><input type="checkbox" checked="true" />Keep Me Logged In</div><button type="submit">Go !</button><a href="#" class="link">Forgot Password</a>
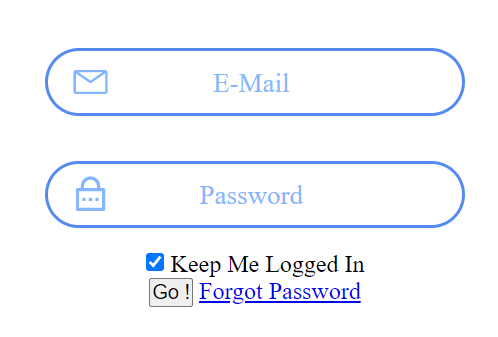
Styling checkbox and keep me text
cssinput[type="checkbox"] {vertical-align: middle;margin-right: 3px;margin-bottom: 3px;font-weight: bold;height: 18px;width: 18px;}.keepme {margin: 15px;color: #71a1bd;font-family: "Scheherazade New", serif;font-weight: bold;}
Styling submit button and forgot password link
cssbutton {width: 260px;height: 45px;left: 1273px;top: 780px;font-family: "Sansation", sans-serif;font-style: normal;font-weight: bold;font-size: 20px;line-height: 28px;cursor: pointer;color: #ffffff;border: none;background: rgba(135, 113, 224, 0.89);border-radius: 25px;}.link {text-align: center;display: block;margin: 18px;color: #71a1bd;font-family: "Scheherazade New", serif;font-weight: bold;text-decoration: none;}
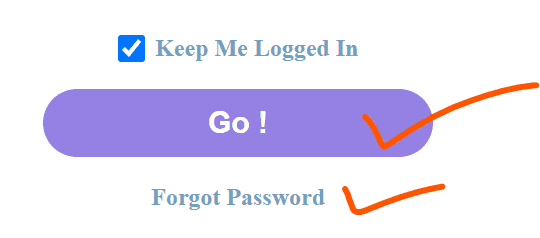
It’s almost done see below 👇
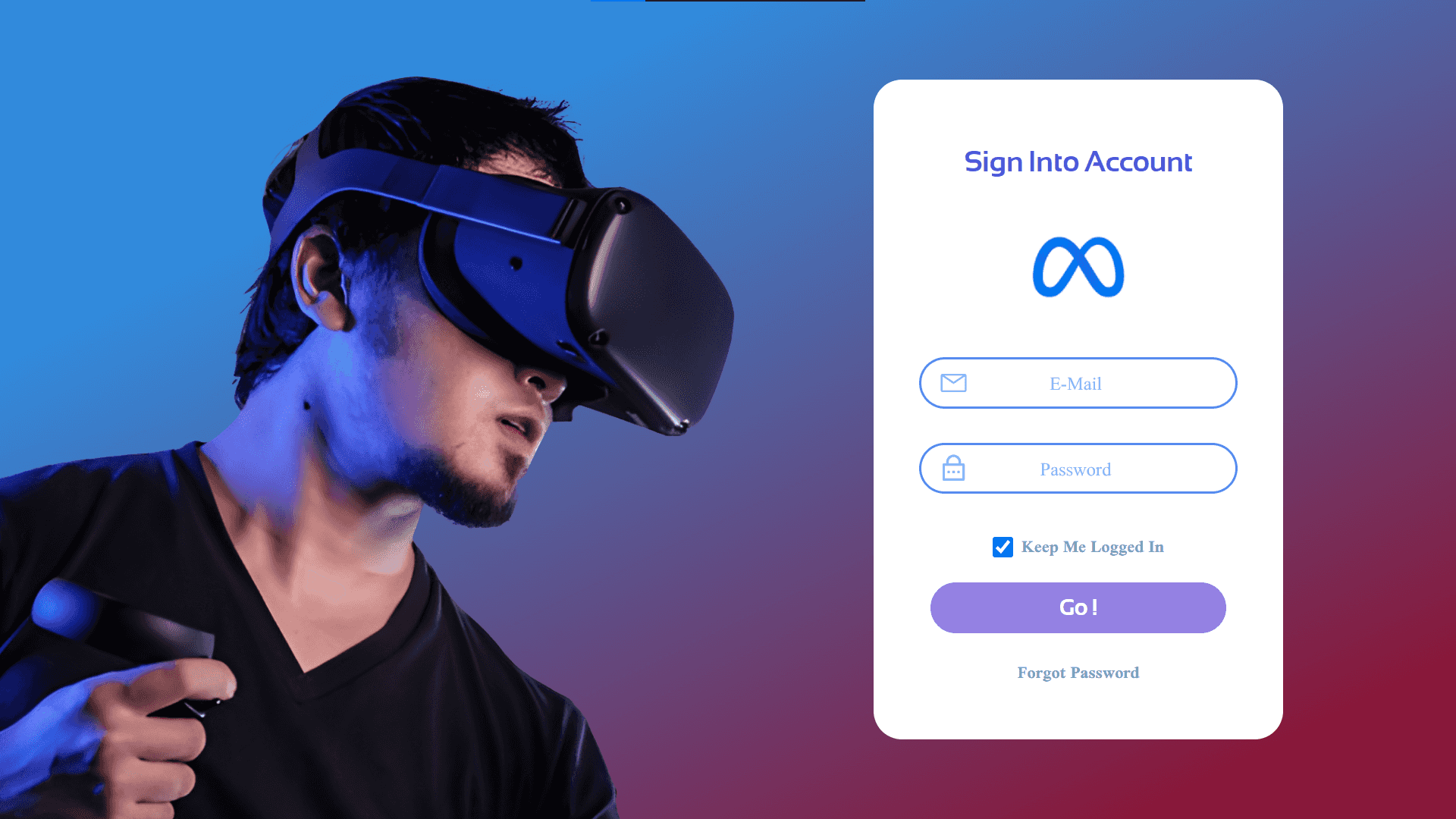
But what about responsiveness ?? 👇
🔗Let's add responsiveness
css@media only screen and (max-width: 700px) {.main-container {background: linear-gradient(138.56deg, #318adc 23.44%, #88183a 89.5%);}.right {left: calc(68% - 500px / 2);}}
In above css code we just defined media query when the size of screen is less than 700px we
- Changed the background of main-container
- Align the right side div from left
Final Output 👇
Congratulation we have successfully created responsive login page 🥳