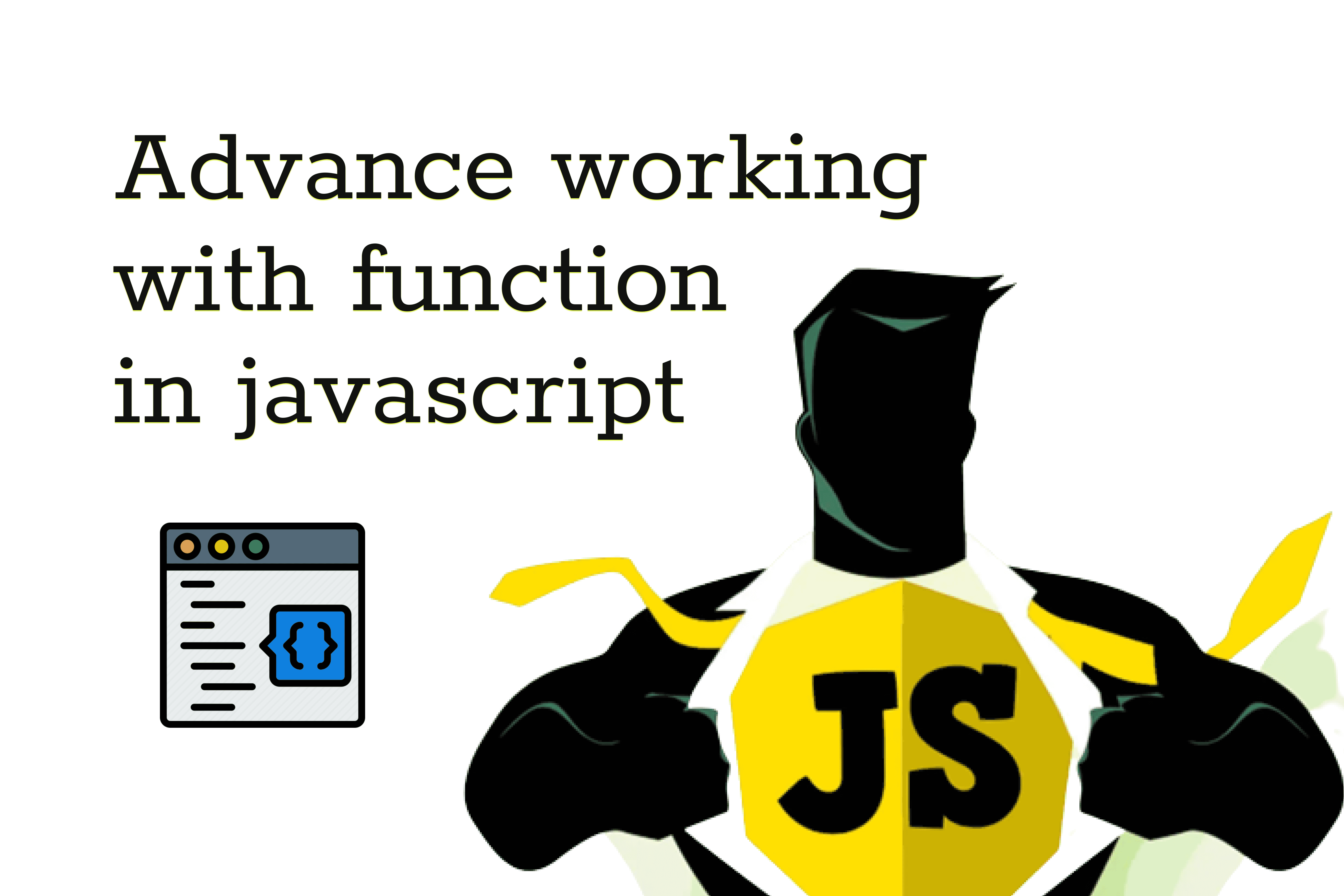
In this post we'll learn advance aspect of working with function in javascript
🔗Recursion
🔗The "new Function”
The “new function”
syntax is another way to create a function. It’s rarely used, but sometimes there’s no alternative.
js// syntaxlet func = new Function([arg1, arg2, ...argN], functionBody);
The function is created with the arguments arg1...argN and the given functionBody.
It’s easier to understand by looking at an example. Here’s a function with two arguments:
jslet sum = new Function("a", "b", "return a + b");console.log(sum(1, 2)); // 3
And here there’s a function without arguments, with only the function body:
jslet msg = new Function('console.log("Hello")');msg(); // Hello
🔗Global object
The global
object provides variables and functions
that are available anywhere
. By default, those that are built into the language or the environment. Recently, globalThis was added to the language, as a standardized name for a global
object, that should be supported across all environments.
All properties of the global object can be accessed directly:
jsxconsole.log("Hello");// is the same aswindow.console.log("Hello");
In a browser, global
functions and variables declared with var (not let/const!)
become the property of the global
object:
jsxvar name = sumit;alert(window.name);// sumit (became a property of the global object)
🔗Function object
In JavaScript, functions are objects.
The different properties include:
- name – the function name. Usually taken from the function definition, but if there’s none, JavaScript tries to guess it from the context (e.g. an assignment).
- length – the number of arguments in the function definition.
If the function is declared as a Function
Expression, and it carries the name, then it is called a Named Function Expression. The name can be used inside to reference itself, for recursive calls or such.
jsxfunction sayHi() {console.log("Hi");}console.log(sayHi.name); // sayHifunction f2(a, b) {}function many(a, b, ...more) {}console.log(f2.length); // 2console.log(many.length); // 2